JScript .NET, Part V: Polymorphism: Multiple Implementations of a Polymorphic Interface - Doc JavaScript
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
JScript .NET, Part V: Polymorphism
Multiple Implementations of a Polymorphic Interface
Polymorphism is easy to show with utility functions. Utility functions are like methods of the main program, or of the global object as it is sometimes called in other languages. You define a utility function in the main program section, before, after, or in between the class definitions. Our utility function, CopyMe()
, accepts an object of type Object
, and returns a copy of it by using the Copy()
method. Here is the utility function:
function CopyMe(ic : Object) : Object { return ic.Copy(); }
The Copy()
method is defined in the interface ICopyObj
, as shown on Page 6:
interface ICopyObj { function Copy() : Object; }
On Page 6 we showed how to implement the Copy()
method for integers. Similarly, we can define it for double precision values:
class CopyDouble implements ICopyObj { public var d : double; public function CopyDouble(d : double) { this.d = d; } public function Copy() : Object { return new CopyDouble(d) } }
The main program section creates four variables. The variable h1
is created by the CopyInt()
constructor. The variable h2
is created by copying h1
with the polymorphic utility CopyMe()
. The variable h3
is created by the CopyDouble()
constructor, and is copied to h4
with the polymorphic utility CopyMe()
. The utility CopyMe()
is polymorphic because it operates on both integer and double values. The programmer who calls CopyMe()
does not care how it is implemented. It is hidden from him. He or she can call CopyMe()
with any object type. We print all variables at the end. Here is the code listing:
// compile with: jsc interfacepoly.js interface ICopyObj { function Copy() : Object; } function CopyMe(ic : Object) : Object { return ic.Copy(); } class CopyInt implements ICopyObj { public var i : int; public function CopyInt(i : int) { this.i = i; } public function Copy() : Object { return new CopyInt(i) } } class CopyDouble implements ICopyObj { public var d : double; public function CopyDouble(d : double) { this.d = d; } public function Copy() : Object { return new CopyDouble(d) } } var h1 : CopyInt = new CopyInt(8); var h2 : CopyInt = CopyMe(h1); var h3 : CopyDouble = new CopyDouble(3.2); var h4 : CopyDouble = CopyMe(h3); print("Original: " + h1.i + " Copy: " + h2.i); print("Original: " + h3.d + " Copy: " + h3.d);
And here is the Command Prompt window, showing the code again (interfacepoly.js
), the jsc
compiler's log messages, and the output of interfacepoly.exe
:
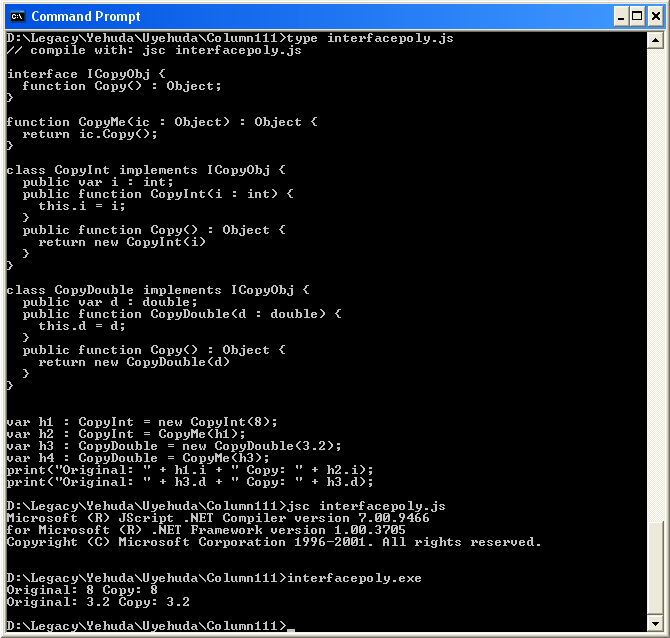
Next: A Final Word
Produced by Yehuda Shiran and Tomer Shiran
All Rights Reserved. Legal Notices.
Created: June 3, 2002
Revised: June 3, 2002
URL: https://www.webreference.com/js/column111/7.html