JScript .NET, Part V: Polymorphism: Implementing a Polymorphic Interface - Doc JavaScript
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
JScript .NET, Part V: Polymorphism
Implementing a Polymorphic Interface
Polymorphism can be and very often is implemented with interfaces. We have shown how to define interfaces in Column 110. Interfaces differ from classes in that they don't include any implementation. You cannot extend an interface. You can only implement an interface by another class. You cannot create objects from an interface, only from classes that implement interfaces. To support polymorphism, define an interface that accepts an object of the type Object
, and implement it in classes that support actual types as integer, double, and user-defined classes. Interface names usually start with I
, to distinguish them from classes.
The following example defines the ICopyObj
interface. It includes one member, Copy()
, which returns a copy of the object of type Object
:
interface ICopyObj { function Copy() : Object; }
The class CopyInt
implements the ICopyObj
interface. It includes one property (i
), and two methods. One method is the class constructor, CopyInt()
. The other method is the interface method, Copy()
:
class CopyInt implements ICopyObj { public var i : int; public function CopyInt(i : int) { this.i = i; } public function Copy() : Object { return new CopyInt(i) } }
The main program creates two variables, h1
and h2
. The variable h1
is generated through the CopyInt()
constructor. The variable h2
is generated through the Copy()
operator. We print both variables at the end. Here is the code listing:
// compile with: jsc interface.js interface ICopyObj { function Copy() : Object; } class CopyInt implements ICopyObj { public var i : int; public function CopyInt(i : int) { this.i = i; } public function Copy() : Object { return new CopyInt(i) } } var h1 : CopyInt = new CopyInt(8); var h2 : CopyInt = h1.Copy(); print("Original: " + h1.i + " Copy: " + h2.i);
And here is the Command Prompt window, showing the code again (interface.js
), the jsc
compiler's log messages, and the output of interface.exe
:
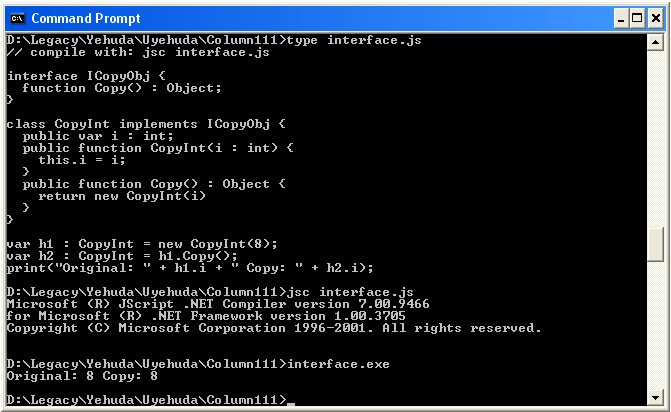
Next: How to write multiple implementations of a polymorphic interface
Produced by Yehuda Shiran and Tomer Shiran
All Rights Reserved. Legal Notices.
Created: June 3, 2002
Revised: June 3, 2002
URL: https://www.webreference.com/js/column111/6.html