JScript .NET, Part V: Polymorphism: Using Derived Objects with Base Methods - Doc JavaScript
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
JScript .NET, Part V: Polymorphism
Using Derived Objects with Base Methods
Let's recap inheritance with an example. It has two classes, Base
and Derived
. The Base
class has one property (an integer, i
) and one method, PrintInBase()
. The Derived
class has one property as well (a double, d
), and one method, PrintInDerived()
.
The main code in JScript .NET is not delimited in any way. You just write the code outside; before, after, or in between the class definitions. The main code in our example allocates two objects, b
of type Base
and d
of type Derived
. We first apply the Base
method (PrintInBase
) to b
. We then apply both the Base
method and the Derived
method to d
. You can see below that the derived object refers to its base class, and can use the base method. In fact, a derived object can refer to any of its base classes. Here is the code:
// compile with: jsc inheritance.js class Base { protected var i : int = 5; public function PrintInBase() : void { print("i is " + i); } } class Derived extends Base { var d : double = 7.3; public function PrintInDerived() : void { print("i is " + i + ", d is " + d); } } var b : Base = new Base(); print("b:"); b.PrintInBase(); var d : Derived = new Derived(); print("d:"); d.PrintInBase(); d.PrintInDerived();
Here is a capture of the command prompt window. It shows the code, inheritance.js
, the messages of the jsc
compiler, and the output of the executable, inheritance.exe
:
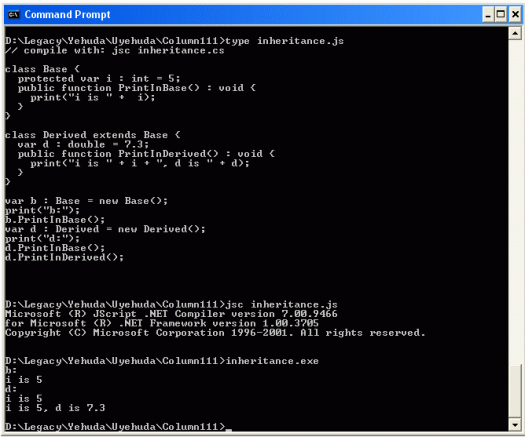
Next: How to use the .NET framework polymorphic methods
Produced by Yehuda Shiran and Tomer Shiran
All Rights Reserved. Legal Notices.
Created: June 3, 2002
Revised: June 3, 2002
URL: https://www.webreference.com/js/column111/3.html