JScript .NET, Part V: Polymorphism: Intrinsic Polymorphic Methods - Doc JavaScript
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
JScript .NET, Part V: Polymorphism
Intrinsic Polymorphic Methods
Polymorphism is considered one of the three cornerstones of object oriented programming languages, besides encapsulation and inheritance. The words "poly" (many) and "morph" (shape) indicate that polymorphism is about supporting many shapes. Polymorphism is based on polymorphic methods. A polymorphic method behaves differently, depending on the object type it is called with. On the outside, all calls to a polymorphic method look identical. The method identifies by itself the type of the object and behaves accordingly. In this way, the nuts and bolts of the code are hidden from you. You just call the object with the operation (method) that you need to apply to the object. You don't care what type the object is of, or how to adapt the operation to the type. The change in behavior is hidden inside the method.
The method ToString()
is a good example. This method is defined for the Object
class that is the base class for every class in the .NET framework (see Page 2). You can use this method for objects that are of type Object
, int
, or double
. You can also redefine this method for a new type of object you create with a new class, without changing the way you call the method.
The following example includes the main program section and a definition of class Base
. We define four variables in the main section: o
, i
, d
, and b
. The variable o
is of type Object
, i
is of type int
, d
is of type double
, and the variable b
is of type Base
. The class Base
has one property (i
), and it redefines the method ToString()
. Instead of just converting the value of the variable to a string, the Base
ToString()
prints a message:
print("i is " + i);
and returns "foo"
. The main program calls ToString()
with each one of the four variables: o
, i
, d
, and b
. The Command Prompt window below shows that all four calls yield the expected results, including the redefined ToString()
method of the Base
class. Here is the code:
// compile with: jsc tostring.js var o : Object = new Object(); var i : int = 9; var d : double = 5.8; var b : Base = new Base(); print("i: " + i.ToString()); print("o: " + o.ToString()); print("d: " + d.ToString()); print("b: " + b.ToString()); class Base { protected var i : int = 5; public function ToString() : String { print("i is " + i); return("foo"); } }
And here is the Command Prompt window, showing the code again (tostring.js
), the jsc
compiler's log messages, and the output of tostring.exe
:
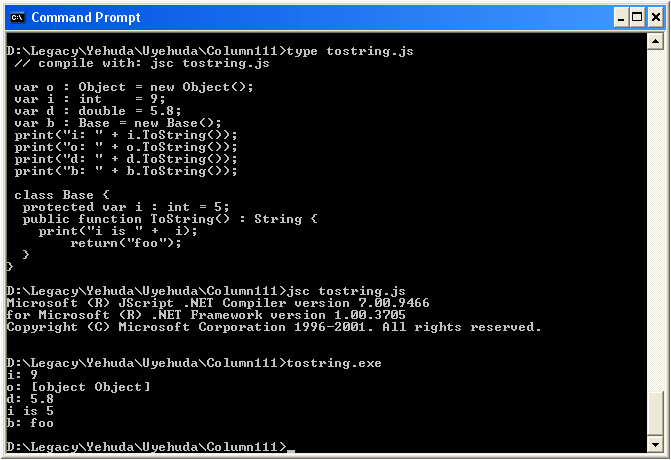
Next: How to write a polymorphic utility function
Produced by Yehuda Shiran and Tomer Shiran
All Rights Reserved. Legal Notices.
Created: June 3, 2002
Revised: June 3, 2002
URL: https://www.webreference.com/js/column111/4.html