JScript .NET, Part V: Polymorphism: Writing a Polymorphic Function - Doc JavaScript
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
JScript .NET, Part V: Polymorphism
Writing a Polymorphic Function
On Page 4 we saw how to use a polymorphic method, ToString()
, and how to redefine it for a new class, Base
. Of course, you can define your own polymorphic methods. All you need to do is define your objects in the most basic type, which is Object
. All types are derived from Object
, so you can pass an integer, a double, and an object of any class to an argument of type Object
.
The function WriteNameValue()
accepts a name and a value as parameters, and prints them. The name is a string and the value is defined of type Object
. In this way, the value parameter can be substituted with any type. The implementation of this utility function is based on the system's polymorphic method, ToString()
. You can write your own implementation, making sure you handle each and every type correctly. Here is the function:
function WriteNameValue(name : String, value : Object) : void { print(name + value.ToString()); }
The rest of the code is very similar to that of Page 4. Instead of calling ToString()
, we call WriteNameValue()
. Here is the code listing:
// compile with: jsc writename.js function WriteNameValue(name : String, value : Object) : void { print(name + value.ToString()); } var o : Object = new Object(); var i : int = 9; var d : double = 5.8; var b : Base = new Base(); WriteNameValue("o: ", o); WriteNameValue("i: ", i); WriteNameValue("d: ", d); WriteNameValue("b: ", b); class Base { protected var i : int = 5; public function ToString() : String { print("i is " + i); return("foo"); } }
And here is the Command Prompt window, showing the code again (writename.js
), the jsc
compiler's log messages, and the output of writename.exe
:
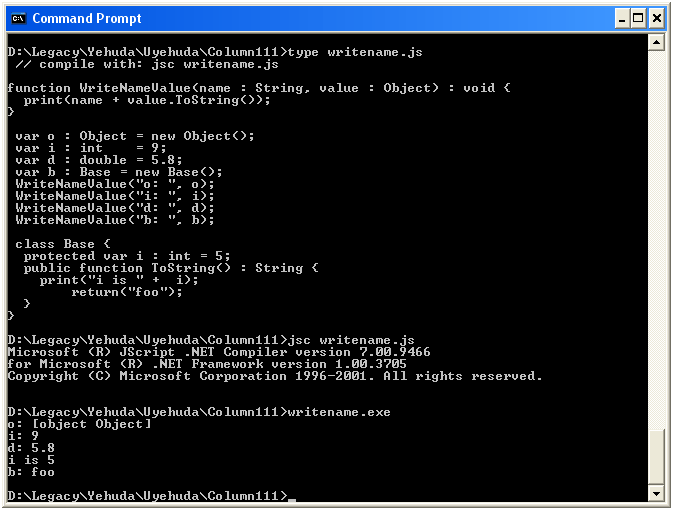
Next: How to implement a polymorphic interface
Produced by Yehuda Shiran and Tomer Shiran
All Rights Reserved. Legal Notices.
Created: June 3, 2002
Revised: June 3, 2002
URL: https://www.webreference.com/js/column111/5.html