Array Power, Part III - DHTML Lab | 13
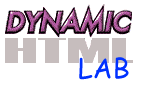
Array Power, Part III
the cnt argument
No cnt Argument
removedElements = myArray.splice(2);
If splice() receives no second argument, it defaults the element count to the number of elements between the first argument pointer and the end of the array.
function array_splice(ind,cnt){ if(arguments.length == 0) return ind; if(typeof ind != "number") ind = 0; if(ind < 0) ind = Math.max(0,this.length + ind); if(ind > this.length) { if(arguments.length > 2) ind = this.length; else return []; } if(arguments.length < 2) cnt = this.length-ind; removeArray = this.slice(ind,ind+cnt); endArray = this.slice(ind+cnt); this.length = ind; for(var i=2;i<arguments.length;i++){ this[this.length] = arguments[i]; } for(var i=0;i<endArray.length;i++){ this[this.length] = endArray[i]; } return removeArray; }
We check for the existence of a second argument. If the argument does not exist, that is, if the length of the function's arguments array is less than 2, we redeclare cnt, assigning it a value equal to the array's length minus the value of ind.
cnt Argument Is Not a Number
removedElements = myArray.splice(2,"cat");
If splice() is incorrectly passed a non-numeric value as a second argument, it defaults the argument to 0.
function array_splice(ind,cnt){ if(arguments.length == 0) return ind; if(typeof ind != "number") ind = 0; if(ind < 0) ind = Math.max(0,this.length + ind); if(ind > this.length) { if(arguments.length > 2) ind = this.length; else return []; } if(arguments.length < 2) cnt = this.length-ind; if (typeof cnt != "number") cnt = 0; removeArray = this.slice(ind,ind+cnt); endArray = this.slice(ind+cnt); this.length = ind; for(var i=2;i<arguments.length;i++){ this[this.length] = arguments[i]; } for(var i=0;i<endArray.length;i++){ this[this.length] = endArray[i]; } return removeArray; }
We check the second argument's (cnt) type. If it is not a number then we redeclare it with a value of 0, before proceeding.
cnt Argument Is a Negative Number
removedElements = myArray.splice(2,-2);
If splice() is passed a negative number as a second argument, it again defaults the argument to 0.
function array_splice(ind,cnt){ if(arguments.length == 0) return ind; if(typeof ind != "number") ind = 0; if(ind < 0) ind = Math.max(0,this.length + ind); if(ind > this.length) { if(arguments.length > 2) ind = this.length; else return []; } if(arguments.length < 2) cnt = this.length-ind; if (typeof cnt != "number") cnt = 0; else cnt = Math.max(0,cnt); removeArray = this.slice(ind,ind+cnt); endArray = this.slice(ind+cnt); this.length = ind; for(var i=2;i<arguments.length;i++){ this[this.length] = arguments[i]; } for(var i=0;i<endArray.length;i++){ this[this.length] = endArray[i]; } return removeArray; }
Using the Math.max() method, we ensure that cnt can never be less than 0.
Small Optimization
The last two statements discussed can be combined into one:
function array_splice(ind,cnt){ if(arguments.length == 0) return ind; if(typeof ind != "number") ind = 0; if(ind < 0) ind = Math.max(0,this.length + ind); if(ind > this.length) { if(arguments.length > 2) ind = this.length; else return []; } if(arguments.length < 2) cnt = this.length-ind; cnt = (typeof cnt == "number") ? Math.max(0,cnt) : 0; removeArray = this.slice(ind,ind+cnt); endArray = this.slice(ind+cnt); this.length = ind; for(var i=2;i<arguments.length;i++){ this[this.length] = arguments[i]; } for(var i=0;i<endArray.length;i++){ this[this.length] = endArray[i]; } return removeArray; }
We now have our complete splice() method prototype.
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: May 16, 2000
Revised: May 16, 2000
URL: https://www.webreference.com/dhtml/column33/9.html