Array Power, Part III - DHTML Lab | 2
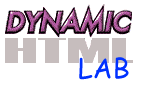
Array Power, Part III
the shift() prototype
The complete prototyping code for splice() looks like this:
if(Array.prototype.splice && typeof([0].splice(0))=="number") Array.prototype.splice = null; if(!Array.prototype.splice) { function array_splice(ind,cnt){ if(arguments.length == 0) return ind; if(typeof ind != "number") ind = 0; if(ind < 0) ind = Math.max(0,this.length + ind); if(ind > this.length) { if(arguments.length > 2) ind = this.length; else return []; } if(arguments.length < 2) cnt = this.length-ind; cnt = (typeof cnt == "number") ? Math.max(0,cnt) : 0; removeArray = this.slice(ind,ind+cnt); endArray = this.slice(ind+cnt); this.length = ind; for(var i=2;i<arguments.length;i++){ this[this.length] = arguments[i]; } for(var i=0;i<endArray.length;i++){ this[this.length] = endArray[i]; } return removeArray; } Array.prototype.splice = array_splice; }
Combining Prototypes
If you use the above code together with the other prototyping code that we have created in these columns, then it can be optimized even more. For example, to append elements to an array, you can use the push() method, given that the push() prototyping code preceeds the splice() code:
if(Array.prototype.push && ([0].push(true)==true)) Array.prototype.push = null; if(Array.prototype.splice && typeof([0].splice(0))=="number") Array.prototype.splice = null; if(!Array.prototype.push) { function array_push() { for(var i=0;i<arguments.length;i++){ this[this.length]=arguments[i] }; return this.length; } Array.prototype.push = array_push; } if(!Array.prototype.splice) { function array_splice(ind,cnt){ if(arguments.length == 0) return ind; if(typeof ind != "number") ind = 0; if(ind < 0) ind = Math.max(0,this.length + ind); if(ind > this.length) { if(arguments.length > 2) ind = this.length; else return []; } if(arguments.length < 2) cnt = this.length-ind; cnt = (typeof cnt == "number") ? Math.max(0,cnt) : 0; removeArray = this.slice(ind,ind+cnt); endArray = this.slice(ind+cnt); this.length = ind; for(var i=2;i<arguments.length;i++){ this.push(arguments[i]); } for(var i=0;i<endArray.length;i++){ this.push(endArray[i]); } return removeArray; } Array.prototype.splice = array_splice; }
On the next page, we have an interactive form demonstrating splice() for both browsers.
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: May 16, 2000
Revised: May 16, 2000
URL: https://www.webreference.com/dhtml/column33/10.html