Low Bandwidth Rollovers: CSS Element Clipping
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
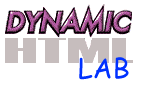
Cutting Your Element Down To Size
2 minutes for clipping
Clipping before Initial Display
CSS allows us to set the visible part of our element, before it is initially displayed, using the clip property:- clip: shape(top right bottom left);
The only shape presently supported is a rectangle. If we wanted to only show a 100x120 pixel portion of our element 20 pixels in from the left and 40 from the top, our declaration would be:
- clip: rect(40 120 140 20);
Dynamic Clipping
In Navigator 4
Clip properties are reflected into Netscape JavaScript as:
- elementName.clip.top,
elementName.clip.right,
elementName.clip.bottom and
elementName.clip.right
To clip our image to show only the part corresponding to the second link, for example, we need only:
- document.elMenu.document.elMenuOver.clip.left = 117;
document.elMenu.document.elMenuOver.clip.right = 181;
We do not need to reference or change clip.top or clip.bottom because they remain constant.
In Explorer 4
Explorer stores an object's clip property value in a single string, retaining the CSS format. For example, if our object's CSS clip declaration was:
- #myObject { clip: rect(40 120 140 20) }
the property document.all.myObject.style.clip would store the string:
- "rect(40px 120px 140px 20px)"
Explorer adds the pixel identifier internally. We do not need to include it if we change the clip value. But, unlike Navigator, Explorer expects all four clip values.
Our second link clip would need this code in Explorer:
- document.all.elMenuOver.style.clip = "rect(0 181 18 117)";
Try it, by placing your mouse anywhere on the menu:
Build the Clipping Script
To make life easier, we need to store the clipping values for the 7 links in a two-dimensional array so that our mapOver function can reference them:
Declare the array:
- arPopups = new Array();
Write a function that creates a second array off each array element
and populates it as necessary. In this way, our code becomes modular
and can be used in other cases with more or fewer elements.
The function accepts three arguments:
which: the link in our image map (an integer from 1 to 7);
from: the left clip value, and
to: the right clip value
- function setBeginEnd(which,from,to) {
arPopups[which] = new Array();
arPopups[which][0] = from;
arPopups[which][1] = to
}
Call the function, taking the clip values from the <AREA> tags:
- setBeginEnd(1,0,116);
setBeginEnd(2,117,181);
setBeginEnd(3,182,222);
setBeginEnd(4,223,263);
setBeginEnd(5,264,339);
setBeginEnd(6,340,397);
setBeginEnd(7,398,468);
To make our Explorer scripting more readable later, we store the constant top and bottom clip values in two variables:
- clTop = 0;
clBot = 18;
In redefining our mapOver function, we must add an additional argument identifying which link should be highlighted (clipped). We will use this argument to reference our array. To minimize the nested ifs in the function, we now check immediately for a mouseout, hide the element and return. If it is a mouseover, we proceed by initializing variables for the left and right clip values, and then check for browser and execute the appropriate script.
- function mapOver(which,on) {
if (IE4) { whichEl = document.all.elMenuOver.style }
else { whichEl = document.elMenu.document.elMenuOver };
if (!on) { whichEl.visibility = "hidden"; return };
clLeft = arPopups[which][0];
clRight = arPopups[which][1];
if (NS4) {
whichEl.clip.left = clLeft;
whichEl.clip.right = clRight;
}
else {
whichEl.clip = "rect(" + clTop + " " + clRight + " " + clBot + " " + clLeft + ")";
}
whichEl.visibility = "visible"
}
And modify your map for the last time, adding values for the new first argument. eg. mapOver(1,true).
- <MAP NAME="mpMenu">
<AREA SHAPE="RECT" COORDS="0,0 116,18" HREF="/"
onMouseOver="mapOver(1,true)" onMouseOut="mapOver(1,false)">
<AREA SHAPE="RECT" COORDS="117,0 181,18" HREF="/contact.php"
onMouseOver="mapOver(2,true)" onMouseOut="mapOver(2,false)">
<AREA SHAPE="RECT" COORDS="182,0 222,18" HREF="https://www.coolcentral.com"
onMouseOver="mapOver(3,true)" onMouseOut="mapOver(3,false)">
<AREA SHAPE="RECT" COORDS="223,0 263,18" HREF="/new/"
onMouseOver="mapOver(4,true)" onMouseOut="mapOver(4,false)">
<AREA SHAPE="RECT" COORDS="264,0 339,18" HREF="/headlines/"
onMouseOver="mapOver(5,true)" onMouseOut="mapOver(5,false)">
<AREA SHAPE="RECT" COORDS="340,0 397,18" HREF="/search.cgi"
onMouseOver="mapOver(6,true)" onMouseOut="mapOver(6,false)">
<AREA SHAPE="RECT" COORDS="398,0 468,18" HREF="/index2.html"
onMouseOver="mapOver(7,true)" onMouseOut="mapOver(7,false)">
</MAP>
The Final Product
Once again, let's take all the code snippets that we have introduced, tighten them up, and see the complete code for our effect:
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: 08/06/97
Revised: 10/16/97
URL: https://www.webreference.com/dhtml/column2/clipping.html