Beginning PHP4 | 7
![]() ![]() ![]() [next] |
Beginning PHP4
Practical Application
It's all very well being able to draw images on the fly, but it's all very superficial. Thousands of Web sites provide copies of the same bland information and links to the same articles/jokes/urban legends. What makes a Web site truly stand out is when you offer something unique and dynamic - something that the people browsing your site can't easily do for themselves or get somewhere else.
What we're going to do for the rest of this chapter is look at how we can take content from a database - content that only you or your client will have - and present it in a way that is clever, useful and appealing for the person browsing your Web site. What we're going to build is an interactive map. By the end of the chapter, we'll have created an application that lets us query a database, and will display a diagram based on the criteria that we have entered.
Interactive Maps
Most of you have had to deal with a map or layout diagram at some time or another. How have you managed to find the road or city that you were looking for? Look it up in an index at the back of the map and then scour the reference it gives you for the one tiny piece of information that you are looking for. What if we could take all of that data, and move it into a database. The index then acts as a search function for our database and the results are highlighted on our map using the graphics techniques that you already know.
The example that we are going to create is a layout diagram for a local shopping mall. Browsers will be able to search for the position of shops by name or single keyword search.
Apart from file format issues, map data can use any number of coordinate systems, ranging from familiar degrees, minutes and seconds to projections like the Universal Transverse Mercator. Each coordinate system would require a different method to reference it to your image map, a topic that's considerably beyond the scope of this book.
We'll be using an existing layout of the shopping mall; from this it will be easy to work out x and y coordinates for the shops, as they will all be based within this one existing image.
When we store the information in our database, we should not only store the coordinate information, but also any extra metadata that we could use to enhance our map. Remember that anybody can get a layout map of an area and hunt their way through it - what is going to add value to our diagram is the fact that we will have data associated with the layout information.
Getting Started
We don't want to have to draw our layout diagram from scratch every single time. PHP has a function that allows us to create an image from an already existing file. What I've done is created the basic outline of the shops in our mall and shown them below. To begin with our mall only has a ground floor. Most malls have some sort of shop naming convention and that is what we have added in the diagrams. The images that we work with in our code will look exactly like these but without the shop names. The shop names will be stored in our database and written onto the diagram by our code.
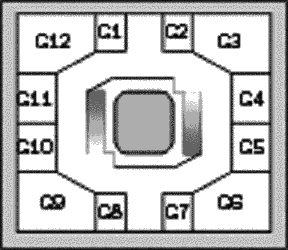
The dark gray blob in the middle represents some foliage, while the rectangles on either side are for up and down escalators. (The owners intend to expand the mall in the future, so we make provision for the escalators now.)
Creating the database
We're going to store all our data in a MySQL database called mapping and use our old friend phpuser (with password phppass) to access this new database:
mysql> create database mapping;
Let's take a look at the layout of our database and the information we'll be storing in it. Below is the rest of our SQL script to create the database:
use mapping;
CREATE TABLE mall (
m_id int(11) DEFAULT '0' NOT NULL AUTO_INCREMENT,
m_floor char(1) NOT NULL,
m_shop varchar(64) NOT NULL,
m_name varchar(64) NOT NULL,
m_phone varchar(64),
m_area varchar(128) NOT NULL,
m_center varchar(64) NOT NULL,
m_desc varchar(128),
PRIMARY KEY (m_id)
);
We have a field called m_id that is the primary key of my table. Because our mall may have more than one level in the future we are storing the level that the shop is on in the m_floor field. Later when we add more floors to our mall this field will be important. m_shop stores the shop name as it is known in the mall layout - this is something like G4 or M8 - we saw these in the figure above. m_name is the name of the shop that the proprietor has given it, m_phone is the shop's phone number. m_area is the important one here as this holds a comma-separated list of the coordinates of each of the corners of the shop's outline. A typical coordinate list will look like this:
500,350,500,500,0,500,0,350,100,350,150,400,350,400,400,350
and consist of alternating x and y coordinates.
The next field m_center is an (x, y) coordinate of the middle of the shop. In order to fill shapes with color we'll have to start filling from somewhere inside the shape, and this center point will be the place where we start.
Possibly a better way to approach this would be to have the script work out the center point itself, saving us the need to store it in the database; however, in order to keep the script as simple as possible and concentrate on the graphical aspects of the script, we'll store the center point for now.
The last field m_desc contains a comma-separated list of keywords describing each shop. This is so that the person browsing through the mall will be able to search for the products or services that they want.
![]() ![]() ![]() [next] |
Revised: March 19, 2001
Created: March 19, 2001