Beginning PHP4 | 9
![]() ![]() ![]() [previous] |
Beginning PHP4
How It Works
After including the include file common_db.inc, we start by creating our blank image canvas and allocating 2 colors to the image:
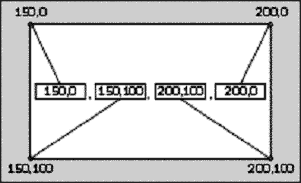
Header("Content-type: image/png");
$image = ImageCreate(501,501);
$white = ImageColorAllocate($image,255,255,255);
$black = ImageColorAllocate($image,0,0,0);
We then connect to the MySQL server on the local machine using the db_connect() function that we defined back in Chapter 11. We set up and execute a SQL query to return all of the m_area fields:
$link_id = db_connect('mapping');
$query = "SELECT m_area FROM mall";
$mallResult = mysql_query($query, $link_id);
We then loop around through the rows that our query returned. The important bits to do with graphics start now.
The first thing we have to do is to grab that comma-separated list of x-y coordinates and turn it into something that we can easily use. The simplest way to do that is to use the explode() function to break up the list (or in this case $mallRow[0]) on commas. We therefore define $area as an array of all of our x and y coordinates:
while ($mallRow = mysql_fetch_array($mallResult)) {
$area = explode(",", $mallRow[0]);
for ($i=0; $i < count($area)-2; $i=$i+2) {
ImageLine($image,$area[$i],$area[$i+1],$area[$i+2],$area[$i+3],$black);
}
ImageLine($image,$area[count($area)-2],
$area[count($area)-1], $area[0], $area[1], $black);
}
The for() statement that we now use may look a little bit strange:
for ($i=0; $i < count($area)-2; $i=$i+2) {
ImageLine($image,$area[$i], $area[$i+1], $area[$i+2], $area[$i+3], $black);
}
What we're doing here is looping around from 0 while $i is less than the count of $area minus 2, each time incrementing $i by 2. The trick to understanding this is that we have an array of alternating x and y coordinates, so we have to use the coordinates in pairs in order to make any sense of them. If $mallRow[0] was:
150,0,150,100,200,100,200,0
then $area would be an array of elements, so the first element $area[0] would be 150, while the last one $area[7] would be 0.
Each pair of elements represents a coordinate set, and our first line should be drawn from the first set to the second. Likewise, the second line will be from the second set to the third and the third line from the third set to the fourth set. The last line is drawn between the last set of coordinates and the first; this completes the shape.
The first time we get into the loop, $i equals 0, so looking at the code that draws the lines:
ImageLine($image, $area[$i], $area[$i+1], $area[$i+2], $area[$i+3], $black);
Our first coordinate set corresponds to the first two elements of the array [$i] and [$i+1]. The second set corresponds to [$i+2] and [$i+3]. The variable $i then has 2 added to its value, and the next pair of coordinates are linked.
The diagram below shows how the loop progresses in steps of 2. The square bracketing on the right (around groups of four elements from $area) show the coordinates that will be used to draw the line in each loop. Remember that our loop is set to continue while $i is less than count($area) -2. Since there are 8 values in the array, it will stop on reaching $i = 6:
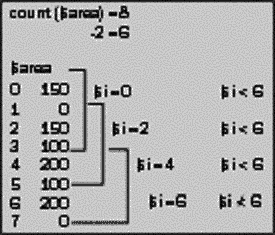
This has only drawn 3 lines for us and the shape will need 4, so once we have dropped out of the bottom of the loop we draw a line from the last pair of elements to the first pair:
ImageLine($image, $area[count($area)-2],
$area[count($area)-1],
$area[0], $area[1], $black);
The last thing we need to do then is output the graphic and clean up memory:
ImagePNG($image);
ImageDestroy($image);
mysql_free_result($mallResult);
?>
![]() ![]() ![]() [previous] |
Revised: March 19, 2001
Created: March 19, 2001