Speeding Up Frame Rates For DHTML Animation in Win98 - DHTML Lab | 6
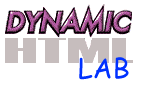
Speeding Up Frame Rates For DHTML Animation in Win98
A Case Study
Speeding Up The Animation Loop With Multithreading
The technique for speeding up the animation loop simply spawns two threads one just after the other. Each thread schedules a call to moveIt() after the minimum 55 ms delay. This increases the number of visible updates within a given time period and doesn't lockout the browser from other events and script. Within the same browser, thread performance varies from less stable animation over the standard single thread method in certain page environments to better animation in others. Between the two browsers, IE performs much better than NN with these multithread speedups.Our initial animation will provide a simple context to start looking at various bi-threaded scripts.
Using setTimeout()
The first bi-threaded script has a coding structure like that of the second single thread setTimeout() animation loop discussed previously but initializes with two successive timeout calls.
function moveIt() { if (!halt) { setTimeout("moveIt()",1); // Element stepping script goes here. } } setTimeout("moveIt()",165); setTimeout("moveIt()",165);
Walking through the script beginning at time 0 for the initially scheduled threads gives:
- t-0:
- Schedule first and second moveIt()
threads in about
165 ms and 165+d ms.
- t~165: THREAD ONE.
- Schedule moveIt()
thread in 55
ms at "t~220".
Increment element position.
Exit thread one and update display. - t~165+d: THREAD TWO.
- Schedule another moveIt()
thread in 55 ms at "t~220+d".
Increment element position.
Exit thread two and update display. - etc...
You can compare the standard 18 Hz, 1 and 2 pixel stepping animations with the 27 Hz, 1 pixel stepping animation using the above technique by clicking below. The 27 Hz rate was measured by noting the time between two distinct events in the animation using the second hand of a wristwatch.
Here are some variations on the same theme that essentially have the same performance as our example.Index each of the two thread calls, have one thread do no rescheduling and have the other thread handle the rescheduling of both of them.
var timerID1, timerID2; function moveIt(thread) { if (!halt) { if (thread==2) { timerID1 = setTimeout("moveIt(1)",1); timerID2 = setTimeout("moveIt(2)",1); } // Element stepping script goes here. } } timerID1 = setTimeout("moveIt(1)",165); timerID2 = setTimeout("moveIt(2)",165);
A couple more possibilities place the setTimeout()'s at the end of the movement script instead of at the beginning of the function as in the above two scripts.
Using setInterval()
With setInterval() one script could look as follows. Note that this script requires browser detection since it will run very erratically on Netscape with delay settings of 1 in both timers, almost locking out the browser. Fortunately delay settings like 50 ms and 75 ms in this method are recognized as actually being 25 ms apart in Navigator and will allow the code to function.
var timerID1, timerID2, delay1, delay2; function moveIt() { if (!halt) { // Element stepping script goes here. } } if (document.all) { delay1=1; delay2=1; } if (document.layers) { delay1=50; delay2=75; } timerID1 = setInterval("moveIt()",delay1); timerID2 = setInterval("moveIt()",delay2);
Analogous to the second
multithread setTimeout() method, one setInterval()
could continuously clear itself
after each iteration while the other takes care of rescheduling.
A hybrid technique can use one setInterval() that's always running and a
setTimeout() that the setInterval() will reschedule.
These speedups ran many times about as smooth as standard methods on IE but
for NN they're more jerky. Since these animations are relatively simple their
performance doesn't necessarily generalize to other situations. Some more
complex and different rendering tasks need to be looked at.
var timerID1, timerID2, delay1, delay2;
function moveIt(thread) {
if (!halt) {
if (thread != 2) clearInterval(timerID1);
else timerID1 = setInterval("moveIt(1)",delay1);
// Element stepping script goes here.
}
}
if (document.all) { delay1=1; delay2=1; }
if (document.layers) { delay1=50; delay2=75; }
timerID1 = setInterval("moveIt(1)",delay1);
timerID2 = setInterval("moveIt(2)",delay2);
var timerID1, timerID2, delay1, delay2;
function moveIt(thread) {
if (!halt) {
if (thread == 2) timerID1 = setTimeout("moveIt(1)",delay1);
// Element stepping script goes here.
}
}
if (document.all) { delay1=1; delay2=1; }
if (document.layers) { delay1=50; delay2=75; }
timerID2 = setInterval("moveIt(2)",delay2);
Produced by Mark Szlazak and
All Rights Reserved. Legal Notices.Created: July 11, 2000
Revised: July 11, 2000
URL: https://www.webreference.com/dhtml/column34/6.html