Array Power, Part III - DHTML Lab | 6
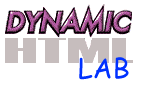
Array Power, Part III
splice()
The splice() method
adds and/or removes elements from an array at any position in the array, and returns a new array of elements removed..
The complete syntax for splice() is:
removedElements = arrayName.splice(index, count, newelement1, newelement2,...,newelementn)
The first argument, index, should be considered mandatory. As we will see later, it may be omitted but it is not good form:
- index
- an integer specifying the position in the array where we will begin to modify it. (required)
- count
- an integer specifying the number of elements to be removed. If count is 0, no elements are removed. If count is omitted, all elements starting with index are removed (optional)
- newelement1, newelement2,...,newelementn
- new elements to be inserted into array. The first element is the third argument of splice(), the second is the fourth argument, and so on. If no elements are listed, splice() will only remove elements. (optional)
- removedElements
- variable representing the array of elements removed from the array. (optional)
Visually, we can represent the splice() method in this way:
Examples
Let's bring our example array, myArray, back into use:
myArray = [0,"cat",true];, or
myArray = new Array(0,"cat",true);
Removing Elements
We want to remove the second element, "cat", from the array, ending up with an array of only two elements:
[0,true]
Using splice(), we can execute this statement:
myArray.splice(1,1);
In plain English, the statement reads:
"Go to index 1 (second element) of myArray and remove 1 element."
If we also want to store this removed element to a variable, myPet, for later use, the syntax would be:
removedElements = myArray.splice(1,1); myPet = removedElements[0];
Remember that splice() always returns an array of elements. If only one element is removed, then an array with a single element is returned. In our example, removedElements looks like this:
["cat"]
To assign any of the removed elements to variables, we must grab them from the returned array, as we do with myPet, above.
Inserting Elements
What if we wanted to insert elements into myArray, instead of removing them? For example, let's insert two new string elements, "dog" and "mouse" after the "cat" element. Our syntax would be:
myArray.splice(2,0,"dog","mouse");
Meaning:
"Go to index 2 (third element) of myArray. Do not remove any elements (0). Insert the elements "dog" and "mouse" at this position."
After statement execution myArray would look like this:
[0,"cat","dog","mouse",true]
Removing and Inserting (Replacing) Elements
We can also replace elements in an array using splice() by removing and inserting elements with a single statement. To get rid of the pesky "cat" element, replacing it with "dog", our splice() method takes this form:
myOldPet = myArray.splice(1,1,"dog");
That is, to say:
"Go to index 1 (second element) of myArray. Remove one element at this position (1). Now insert the element "dog" at this position. Assign the removed element to the myOldPet array."
myArray would then look like this:
[0,"dog",true]
Now, how can we achieve the same functionality of the three examples above without the splice() method? That is, how can we do it in Explorer?.
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: May 16, 2000
Revised: May 16, 2000
URL: https://www.webreference.com/dhtml/column33/2.html