Array Power, Part III - DHTML Lab | 5
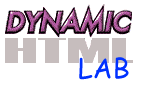
Array Power, Part III
arrayprototype.js
arrayprototype.js collects all the code discussed in the three articles in this series into one external file.
Load the file early in your Web page to make the prototyped methods available to all your scripts.
This file may be downloaded in ZIP format.
Important Note:As discussed, Navigator loads different behavior for push() and splice() depending on the value of the LANGUAGE= attribute. If you omit it or use the same one, ex."JavaScript1.2", all the time, then arrayprototype.js will function as expected. If you mix the attribute values in your script, for example:
<SCRIPT LANGUAGE="JavaScript" SRC="arrayprototype.js" TYPE="text/javascript"></SCRIPT> <SCRIPT LANGUAGE="JavaScript1.2" TYPE="text/javascript"> ...statements... </SCRIPT>
then arrayprototype.js will not function as expected on newer versions of Navigator. In the code, above, new versions of Navigator will load JavaScript 1.3 (default) for the external file, find that the methods have the correct behavior, and not prototype them. Later when you execute the JavaScript 1.2 script, the unwanted old behavior will kick in.
To always get the correct behavior and not have to worry about the value of LANGUAGE=, load the external file as a JavaScript 1.2 file. This will force prototyping and desired behavior:
<SCRIPT LANGUAGE="JavaScript1.2" SRC="arrayprototype.js" TYPE="text/javascript"></SCRIPT>
/* arrayprototype.js * by Peter Belesis. v1.0 000516 * Copyright (c) 2000 Peter Belesis. All Rights Reserved. * Originally published and documented at https://www.dhtmlab.com/ * License to use is granted if and only if this entire copyright notice * is included. * This program is free software; you can redistribute it and/or modify * it under the terms of the GNU General Public License as published by * the Free Software Foundation; either version 2 of the License, or * (at your option) any later version. */ if(Array.prototype.push && ([0].push(true)==true))Array.prototype.push = null; if(Array.prototype.splice && typeof([0].splice(0))=="number")Array.prototype.splice = null; if(!Array.prototype.shift) { function array_shift() { firstElement = this[0]; this.reverse(); this.length = Math.max(this.length-1,0); this.reverse(); return firstElement; } Array.prototype.shift = array_shift; } if(!Array.prototype.unshift) { function array_unshift() { this.reverse(); for(var i=arguments.length-1;i>=0;i--){ this[this.length]=arguments[i] } this.reverse(); return this.length } Array.prototype.unshift = array_unshift; } if(!Array.prototype.push) { function array_push() { for(var i=0;i<arguments.length;i++){ this[this.length]=arguments[i] }; return this.length; } Array.prototype.push = array_push; } if(!Array.prototype.pop) { function array_pop(){ lastElement = this[this.length-1]; this.length = Math.max(this.length-1,0); return lastElement; } Array.prototype.pop = array_pop; } if(!Array.prototype.splice) { function array_splice(ind,cnt){ if(arguments.length == 0) return ind; if(typeof ind != "number") ind = 0; if(ind < 0) ind = Math.max(0,this.length + ind); if(ind > this.length) { if(arguments.length > 2) ind = this.length; else return []; } if(arguments.length < 2) cnt = this.length-ind; cnt = (typeof cnt == "number") ? Math.max(0,cnt) : 0; removeArray = this.slice(ind,ind+cnt); endArray = this.slice(ind+cnt); this.length = ind; for(var i=2;i<arguments.length;i++){ this[this.length] = arguments[i]; } for(var i=0;i<endArray.length;i++){ this[this.length] = endArray[i]; } return removeArray; } Array.prototype.splice = array_splice; } //end
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: May 16, 2000
Revised: May 16, 2000
URL: https://www.webreference.com/dhtml/column33/13.html