Array Power, Part II - DHTML Lab | 4
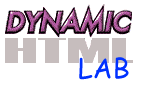
Array Power, Part II
prototyping push()
Array sniffing
Let's create an array with a single element. The element is the number 0. This is as short an array as we can declare:
myArray = [0];
Now we push a non-numeric value, like the Boolean true, onto myArray:
returnValue = myArray.push(true);
If the JavaScript interpreter complies with JavaScript 1.2, then push() will return the last element in the array (the true value.) If the interpreter is more recent, then push() will return the length of the array. The following statements identify the interpreter:
myArray = [0]; oldInterpreter = (myArray.push(true)==true)
We can shorten the code, by omitting the array declaration statement, and using an array literal instead:
oldInterpreter = ([0].push(true)==true)
So, we know how to identify an old JavaScript interpreter. Now we need to expand our statement to achieve our goal, which is:
Check if push() is supported. If it is, check which behavior of push() is supported. If the old behavior is supported, then disable the old support.
if (Array.prototype.push && ([0].push(true)==true)) Array.prototype.push = null;
In plain English, the above statement reads:
If the push() method exists and if the element pushed onto the array is returned from the method, then we have an old interpreter, which means that we should disable the push() method completely by assigning a null value to it.
Prototyping a New push() Method
Unlike the previous methods that we prototyped, the push() prototyping code is available, not only to Explorer, but to versions of Navigator that have the old push() method, and to scripts running under LANGUAGE=JavaScript1.2. In other words, when we have finished prototyping push() all instances of push() will behave the same way regardless of the browser environment:
if (Array.prototype.push && ([0].push(true)==true)) Array.prototype.push = null; if(!Array.prototype.push) { function array_push() { for(i=0;i<arguments.length;i++){ this[this.length] = arguments[i]; } return this.length; } Array.prototype.push = array_push; }
We first check Array.prototype for the existence of an push() method. If it does not exist, we proceed to create one (Explorer and JS1.2 behavior in Navigator). If it exists we do nothing (Navigator JS1.3 behavior):
if(!Array.prototype.push) { . execute these statements only if push() does not exist . }
The function that represents the new push() method is called array_push() and this function is assigned to the push method of Array.prototype, making it available to all arrays in both browsers.
Using our example,
arLength = myArray.push("dog","mouse");
the function would proceed as follows when called:
Statement used | myArray becomes | |
before method call | [0,"cat",true] | |
1. | The function loops through all the arguments passed to it:
for(i=0;i<arguments.length;i++){}; | |
2. | Each argument is appended to the array: this[this.length] = arguments[i]; | [true,"cat",0,"dog"] [true,"cat",0,"dog","mouse"] |
3. | The length of the array is returned: return this.length; |
On the next page, we have an interactive form demonstrating push() for both browsers.
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: May 2, 2000
Revised: May 2, 2000
URL: https://www.webreference.com/dhtml/column32/4.html