Dynamic Images: the full code
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
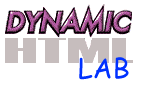
Dynamic Images:
SPECIAL EDITION; the director's cut
Unlike in our previous columns, we did not build a single piece of code page-by-page. Every page was its own little routine. It is fairly straightforward to put it all together, however. We promised at the onset that we would create a routine to be appended to already existing documents. The routines in the previous pages were for in-page inclusion.
Combining Into One File
As our code has developed, we have two obstacles to the single file idea. The CSS element declaration in our HEAD and the SCRIPT at the end of our document.
First, let's combine the two, by using an in-line STYLE declaration. Our little end snippet then becomes:
- if (ver4) {
document.write("<DIV ID='elZoom' STYLE='position: absolute; visibility: hidden'></DIV>");
if (IE4) { document.elZoom = document.all.elZoom.style }
}
Our whole JavaScript code could be placed at the end of the document, as it only contains functions to be used after the page has loaded. Therefore, all our script could be at the end with the snippet appended to it. Since it's all in one place now, we might as well place it in an external file. Therefore, the end of our HTML looks like this:
- <SCRIPT LANGUAGE="JavaScript1.1" SRC="zoomOut.js"></SCRIPT>
</BODY>
</HTML>
The File
We've added variables for all the options. In this version of zoomOut.js only one of the options can be selected at a time. If more than one is selected, it will only see the second one. Don't forget that inWind is special and can be used in combination with any of the others.
Using different options on different pages
If you want to link to the same file from different pages, and have different options for each page activated, it is simple. In zoomOut.js, make sure all variables are false or null. Then immediately following the insertion point in your page, put in a little SCRIPT to set the variables. For example, to show only JPGs in-window, with a 3.2 zoom out:
- <SCRIPT LANGUAGE="JavaScript1.1" SRC="zoomOut.js"></SCRIPT>
<SCRIPT LANGUAGE="JavaScript1.1">
<!-- inWind = true; justJPGs = true; scale = 3.2; -->
</SCRIPT>
</BODY>
</HTML>
zoomOut.js
IE4 = (document.all) ? 1 : 0; NS4 = (document.layers) ? 1 : 0; ver4 = (IE4 || NS4) ? 1 : 0; if (ver4) { whichIm = null; zoomed = false; gotIt = false; scale = 2.5; allPics = false; noLinks = false; justGIFs = false; justJPGs = false; byName = false; inWind = false; useName = null; if (NS4) { document.captureEvents(Event.MOUSEDOWN); document.onmousedown = findIt; } else { document.onclick = findIt } } function findIt(e) { if (zoomed) { zoomIn(); return false; } if (IE4) { isImage = (event.srcElement.tagName == "IMG") ? 1 : 0; if (!isImage) { return true } whichIm = event.srcElement; isAnchor = (noLinks && event.srcElement.parentElement.tagName == "A") ? 1 : 0; isLink = (isAnchor && event.srcElement.parentElement.href) ? 1 : 0; } else { if (e.target=="[object Image]") { whichIm = e.target; isLink = false; gotIt = true; } else { isLink = true; l = e.pageX; t = e.pageY; gotIt = getImage(l,t) } if (!gotIt) { return true }; isImage = true; } isGIF = (justGIFs && whichIm.src.indexOf(".gif") != -1) ? 1 : 0; isJPG = ((justJPGs && whichIm.src.indexOf(".jpg") != -1) || (justJPGs && whichIm.src.indexOf(".jpeg")!=-1)) ? 1 : 0; isName = (byName && whichIm.name && whichIm.name.indexOf(useName) != -1) ? 1 : 0; isOK = (allPics) ? 1 : 0; if (justGIFs) { isOK = (isGIF) ? 1 : 0 }; if (justJPGs) { isOK = (isJPG) ? 1 : 0 }; if (justGIFs && justJPGs) { isOK = (isGIF || isJPG) ? 1 : 0 }; if (noLinks) { isOK = (!isLink) ? 1 : 0 }; if (byName) { isOK = (isName) ? 1 : 0 }; if (isOK) { if (IE4) { if (inWind) { zoomOutInEl() } else { zoomOutInPage() }; return false; } else { zoomOutInEl(); return false } } return true } function getImage(l,t) { for (i=0; i<document.images.length; i++) { imX1 = document.images[i].x; imX2 = imX1 + document.images[i].width; imY1 = document.images[i].y; imY2 = imY1 + document.images[i].height; if ((l >= imX1 && l <= imX2) && (t >= imY1 && t<= imY2)) { whichIm = document.images[i]; gotIt = true; break; } } return gotIt } function zoomOutInPage() { whichIm.width = whichIm.width * scale; whichIm.height = whichIm.height * scale; zoomed = true; } function zoomOutInEl(){ newWidth = whichIm.width * scale; newHeight = whichIm.height * scale; bigImStr = "<IMG NAME='imBig' SRC=\"" + whichIm.src + "\" WIDTH=" + newWidth + " HEIGHT=" + newHeight + " BORDER=0>"; if (NS4) { with (document.elZoom.document) { open(); write(bigImStr); close(); } } else { elZoom.innerHTML = bigImStr }; if (NS4) { document.elZoom.moveTo(whichIm.x,whichIm.y); winPosL = document.elZoom.left - pageXOffset; winPosT = document.elZoom.top - pageYOffset; } else { document.elZoom.left = whichIm.offsetLeft + whichIm.hspace; document.elZoom.top = whichIm.offsetTop; winPosL = elZoom.offsetLeft - document.body.scrollLeft; winPosT = elZoom.offsetTop - document.body.scrollTop; } winWidth = (NS4) ? window.innerWidth : document.body.clientWidth; winHeight = (NS4) ? window.innerHeight : document.body.clientHeight; if (winPosL + newWidth > winWidth) { newPosL = (winWidth - (winPosL + newWidth) - 30); document.elZoom.left = parseInt(document.elZoom.left) + newPosL; } if (winPosT + newHeight > winHeight) { newPosT = (winHeight - (winPosT + newHeight) - 10); document.elZoom.top = parseInt(document.elZoom.top) + newPosT; } document.elZoom.visibility = "visible"; zoomed = true; } function zoomIn() { if (IE4 && !inWind) { whichIm.width = whichIm.width/scale; whichIm.height = whichIm.height/scale; } else { document.elZoom.visibility='hidden' } gotIt = false; zoomed = false; } if (ver4) { document.write("<DIV ID='elZoom' STYLE='position: absolute; visibility: hidden;'></DIV>"); if (IE4) { document.elZoom = document.all.elZoom.style } }
Download
This file is, of course, already in your cache, since it was used with many of the previous pages. But you can download it if you like.
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: 08/27/97
Revised: 11/09/97
URL: https://www.webreference.com/dhtml/column3/allCode.html