DHTML Lab - dhtmlab.com - Smooth animation using DHTML | 8
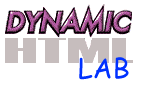
Smooth animation using DHTML
part 5 - myDelay()
I didn't simply stop testing things once I had seen the initial results from using setTimeout()
and setInterval()
. Since I got lackluster performance under Windows 98 I wanted to look for other ways of doing the animation. Not necessarily a better way, but a way that would give more control of the animation speed.
The result was my own delay function. JavaScript doesn't have any delay methods/functions available, so I had to write my own. When people drop by comp.lang.javascript and ask for a delay function the answer is usually "Write your script so it can use setTimeout()
." As I had already found out, setTimeout()
couldn't help me. Regardless of what I ended up with doing, I had to keep setInterval()
and setTimeout()
out of the code.
I ended up with a while()
-loop that would use the Date()
object for time checks. The code is very simple, but it's not a nice thing to do. It locks the browser as long as the while()
-loop runs, but for testing purposes that would have to suffice.
<SCRIPT LANGUAGE="JavaScript1.2" TYPE="text/javascript"> function myDelay(myTime) { rightNow = new Date(); var now = new Date(); now.setTime(now.getTime() + myTime); while(rightNow < now) { rightNow = new Date(); } } </SCRIPT>
As you can see the function creates two Date()
objects, sets one of them to a preset time in the future (now + the delay) and then the while()
-loop runs until the time matches the set time. Very simple, very rough, it works, but it's not a really good solution. Try it out in the test section if you want to.
Regardless of the actual usefulness of the delay code I wanted to test it. Just out of curiosity, actually. It looked promising at first, but proved to be totally useless once it got tested. There are several problems with the solution. First of all, it didn't work on several of the test systems. One of the goals of these tests have been to find a cross-browser cross-platform solution, and since myDelay()
fails here it's of course useless.
Secondly, you would have to use a for()
-loop to do the movement. The loop would first call myMoveTo()
and then call myDelay()
which would result in a delayed myMoveTo()
. As I've noted earlier the for()
-loop does not produce any visible movement in MSIE. If you'd want to get visible movement in MSIE you'd have to use either setTimeout()
or setInterval()
, and as I've already mentioned I cannot use either of those.
The delay-function actually worked under Windows 98. Since it produces no visible movement in MSIE, and didn't run any faster than the other solutions I've tested, the result is that MSIE "hangs" for 80 seconds. Not at all useful or nice to your users, but it could be a good prank on your friends.
It's time to draw some conclusions from these tests.
Produced by Morten Wang and
All Rights Reserved. Legal Notices.Created: Jan 03, 2000
Revised: Jan 03, 2000
URL: https://www.webreference.com/dhtml/column28/part-5.html