DHTML Lab: Cross-Browser Visibility Transitions; The Complete Code 2/2
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
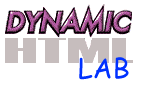
Cross-Browser Visibility Transitions
SPECIAL EDITION; the director's cut 2/2
The External Script (trans.js)
This file may be download in ZIP format
Cross-browser code is blue, Navigator-specific code is red, and Explorer code is green.
/*trans.js (Cross-browser Visibility Transitions) * Copyright (c) 1999-2000 internet.com Corp. To receive the right to license * this code to use on your site the original code must be copied from * Webreference.com. License is granted if and only if this entire copyright * notice is included, and you link from the page on which the code is used * to Webreference at https://webreference.com/dhtml/ for the latest version. * By Peter Belesis. v1.0 980427 - column 19 */ function doTrans(el,transNo,dur) { whichEl = (IE4) ? eval(el) : eval("document." + el); transNo = parseInt(transNo); arHidden = new Array(1,3,14,16); if (IE4) {doTransIE(whichEl,transNo,dur)} else {doTransNS(whichEl,transNo,dur)} } function doTransIE(whichEl,transNo,dur) { whichEl.style.filter = "revealTrans(duration=" + dur + ",transition=" + transNo + ")"; endState = "hidden"; for (i=0; i<arHidden.length; i++) { if (arHidden[i] == transNo) { endState = "visible"; whichEl.style.visibility="hidden"; } } whichEl.onfilterchange = clearFilt; whichEl.filters.revealTrans.apply(); whichEl.style.visibility = endState; whichEl.filters.revealTrans.play(); } function clearFilt() { whichEl.style.filter = ""; } function doTransNS(whichEl,transNo,dur) { whichEl.origLeft = whichEl.clip.left; whichEl.origTop = whichEl.clip.top; whichEl.origRight = whichEl.clip.right; whichEl.origBottom = whichEl.clip.bottom; whichEl.hideIt = hideIt; mSecs = dur * 1000; intval = 100; visits = (mSecs/intval); fullW = whichEl.clip.width; fullH = whichEl.clip.height;; halfW = fullW/2; halfH = fullH/2; alternateW = alternateH = true; switch (transNo) { case 0: case 12: incW = halfW/visits; incrementW = (incW >= 1) ? parseInt(incW) : 0; xtraPixW = Math.round((incW - incrementW) * visits); incH = halfH/visits; incrementH = (incH >= 1) ? parseInt(incH) : 0; xtraPixH = Math.round((incH - incrementH) * visits); whichEl.transFunct = zero; break; case 1: incW = halfW/visits; incrementW = (incW >= 1) ? parseInt(incW) : 0; xtraPixW = Math.round((incW - incrementW) * visits); incH = halfH/visits; incrementH = (incH >= 1) ? parseInt(incH) : 0; xtraPixH = Math.round((incH - incrementH) * visits); whichEl.clip.left += halfW; whichEl.clip.right -= halfW; whichEl.clip.top += halfH; whichEl.clip.bottom -= halfH; whichEl.visibility = "show"; whichEl.transFunct = one; break; case 2: largest = Math.max(halfW,halfH); increment = Math.round(largest/visits); largerW = (halfW > halfH); whichEl.transFunct = two; break; case 3: largest = Math.max(halfW,halfH); increment = Math.round(largest/visits); largerW = (halfW > halfH); whichEl.clip.left += halfW; whichEl.clip.right -= halfW; whichEl.clip.top += halfH; whichEl.clip.bottom -= halfH; whichEl.visibility = "show"; whichEl.transFunct = three; break; case 4: increment = fullH/visits; whichEl.transFunct = four; break; case 5: increment = fullH/visits; whichEl.transFunct = five; break; case 6: increment = fullW/visits; whichEl.transFunct = six; break; case 7: increment = fullW/visits; whichEl.transFunct = seven; break; case 8: case 10: case 13: case 22: increment = halfW/visits; whichEl.transFunct = thirteen; break; case 9: case 11: case 15: case 21: increment = halfH/visits; whichEl.transFunct = fifteen; break; case 14: increment = halfW/visits; whichEl.clip.left += halfW; whichEl.clip.right -= halfW; whichEl.visibility = "show"; whichEl.transFunct = fourteen; break; case 16: increment = halfH/visits; whichEl.clip.top += halfH; whichEl.clip.bottom -= halfH; whichEl.visibility = "show"; whichEl.transFunct = sixteen; break; case 17: incW = fullW/visits; incrementW = (incW >= 1) ? parseInt(incW) : 0; xtraPixW = Math.round((incW - incrementW) * visits); incH = fullH/visits; incrementH = (incH >= 1) ? parseInt(incH) : 0; xtraPixH = Math.round((incH - incrementH) * visits); whichEl.transFunct = seventeen; break; case 18: incW = fullW/visits; incrementW = (incW >= 1) ? parseInt(incW) : 0; xtraPixW = Math.round((incW - incrementW) * visits); incH = fullH/visits; incrementH = (incH >= 1) ? parseInt(incH) : 0; xtraPixH = Math.round((incH - incrementH) * visits); whichEl.transFunct = eighteen; break; case 19: incW = fullW/visits; incrementW = (incW >= 1) ? parseInt(incW) : 0; xtraPixW = Math.round((incW - incrementW) * visits); incH = fullH/visits; incrementH = (incH >= 1) ? parseInt(incH) : 0; xtraPixH = Math.round((incH - incrementH) * visits); whichEl.transFunct = nineteen; break; case 20: incW = fullW/visits; incrementW = (incW >= 1) ? parseInt(incW) : 0; xtraPixW = Math.round((incW - incrementW) * visits); incH = fullH/visits; incrementH = (incH >= 1) ? parseInt(incH) : 0; xtraPixH = Math.round((incH - incrementH) * visits); whichEl.transFunct = twenty; break; case 23: twentythree(dur); return; } transTimer = setInterval("whichEl.transFunct()",intval) } /* transitions 0 & 12: 0 - BOX IN - behavior exactly as IE 12 - RANDOM DISSOLVE - not possible, transition 0 substituted element transition from visible to hidden */ function zero() { if (xtraPixW > 0) { if (xtraPixW <= visits) { if (alternateW) { this.clip.left++; this.clip.right--; xtraPixW--; } alternateW = !alternateW; } else { this.clip.left++; this.clip.right--; xtraPixW--; } } this.clip.left += incrementW; this.clip.right -= incrementW; if (xtraPixH > 0) { if (xtraPixH <= visits) { if (alternateH) { this.clip.top++; this.clip.bottom--; xtraPixH--; } alternateH = !alternateH; } else { this.clip.top++; this.clip.bottom--; xtraPixH--; } } this.clip.top += incrementH; this.clip.bottom -= incrementH; visits--; if (this.clip.height <= 0 && this.clip.width <= 0) { clearInterval(transTimer); this.hideIt(); } } /* transition 1 - BOX OUT: behavior exactly as IE; element transition from hidden to visible; */ function one(){ if ((this.clip.left - this.origLeft) <= incrementW) { this.clip.left = this.origLeft; this.clip.right = this.origRight; } else { if (xtraPixW > 0) { if (xtraPixW <= visits) { if (alternateW) { this.clip.left--; this.clip.right++; xtraPixW--; } alternateW = !alternateW; } else { this.clip.left--; this.clip.right++; xtraPixW--; } } this.clip.left -= incrementW; this.clip.right += incrementW; } if ((this.clip.top - this.origTop) <= incrementH) { this.clip.top = this.origTop; this.clip.bottom = this.origBottom; } else { if (xtraPixH > 0) { if (xtraPixH <= visits) { if (alternateH) { this.clip.top--; this.clip.bottom++; xtraPixH--; } alternateH = !alternateH; } else { this.clip.top--; this.clip.bottom++; xtraPixH--; } } this.clip.top -= incrementH; this.clip.bottom += incrementH; } visits--; if (this.clip.left==this.origLeft && this.clip.top==this.origTop) clearInterval(transTimer); } /* transition 2 - CIRCLE IN: circle shape not possible; square substituted; element transition from visible to hidden; */ function two(){ if (largerW) { this.clip.left += increment; this.clip.right -= increment; if (this.clip.height >= this.clip.width) { this.clip.top += increment; this.clip.bottom -= increment; } } else { this.clip.top += increment; this.clip.bottom -= increment; if (this.clip.width >= this.clip.height){ this.clip.left += increment; this.clip.right -= increment;} } if (this.clip.height<=0 && this.clip.width<=0) { clearInterval(transTimer); this.hideIt(); } } /* transition 3 - CIRCLE OUT: circle shape not possible; square substituted; element transition from hidden to visible; */ function three(){ if (largerW) { if ((this.clip.left - this.origLeft) <= increment) { this.clip.left = this.origLeft; this.clip.right = this.origRight; } else { this.clip.left -= increment; this.clip.right += increment; } if (this.clip.height <= this.clip.width) { if ((this.clip.top - this.origTop) <= increment) { this.clip.top = this.origTop; this.clip.bottom = this.origBottom; } else { this.clip.top -= increment; this.clip.bottom += increment; } } } else { if ((this.clip.top - this.origTop) <= increment) { this.clip.top = this.origTop; this.clip.bottom = this.origBottom; } else { this.clip.top -= increment; this.clip.bottom += increment; } if (this.clip.width <= this.clip.height) { if ((this.clip.left - this.origLeft) <= increment) { this.clip.left = this.origLeft; this.clip.right = this.origRight; } else { this.clip.left -= increment; this.clip.right += increment; } } } if (this.clip.left == this.origLeft && this.clip.top == this.origTop) clearInterval(transTimer); } /* transition 4 - WIPE UP: behavior exactly as IE; element transition from visible to hidden; */ function four(){ this.clip.height -= increment; if (this.clip.bottom <= 0) { clearInterval(transTimer); this.hideIt(); } } /* transition 5 - WIPE DOWN: behavior exactly as IE; element transition from visible to hidden; */ function five(){ this.clip.top += increment; if (this.clip.height <= 0) { clearInterval(transTimer); this.hideIt(); } } /* transition 6 - WIPE RIGHT: behavior exactly as IE; element transition from visible to hidden; */ function six(){ this.clip.left += increment; if (this.clip.width <= 0) { clearInterval(transTimer); this.hideIt(); } } /* transition 7 - WIPE LEFT: behavior exactly as IE; element transition from visible to hidden; */ function seven(){ this.clip.right -= increment; if (this.clip.width <= 0) { clearInterval(transTimer); this.hideIt(); } } /* transitions 8, 10, 13 & 22: 8 - VERTICAL BLINDS - not possible, transition 13 substituted; 10 - CHECKERBOARD ACROSS - not possible, transition 13 substituted; 13 - SPLIT VERTICAL IN - behavior exactly as IE; 22 - RANDOM BARS VERTICAL - not possible, transition 13 substituted; element transition from visible to hidden; */ function thirteen(){ this.clip.left += increment; this.clip.right -= increment; if (this.clip.width <= 0) { clearInterval(transTimer); this.hideIt(); } } /* transitions 9, 11, 15 & 21: 9 - HORIZONTAL BLINDS - not possible, transition 15 substituted; 11 - CHECKERBOARD DOWN - not possible, transition 15 substituted; 15 - SPLIT HORIZONTAL IN - behavior exactly as IE; 21 - RANDOM BARS HORIZONTAL - not possible, transition 15 substituted; element transition from visible to hidden; */ function fifteen(){ this.clip.top += increment; this.clip.bottom -= increment; if (this.clip.height <= 0) { clearInterval(transTimer); this.hideIt(); } } /* transition 14 - SPLIT VERTICAL OUT: behavior exactly as IE; element transition from hidden to visible; */ function fourteen(){ if ((this.clip.left - this.origLeft) <= increment) { this.clip.left = this.origLeft; this.clip.right = this.origRight; clearInterval(transTimer); } else { this.clip.left -= increment; this.clip.right += increment; } } /* transition 16 - SPLIT HORIZONTAL OUT: behavior exactly as IE; element transition from hidden to visible; */ function sixteen(){ if ((this.clip.top - this.origTop) <= increment) { this.clip.top = this.origTop; this.clip.bottom = this.origBottom; clearInterval(transTimer); } else { this.clip.top -= increment; this.clip.bottom += increment; } } /* transition 17 - STRIPS LEFT DOWN: diagonal wipe not possible; box-in to bottom-left corner substituted; element transition from visible to hidden; */ function seventeen(){ if (xtraPixW > 0) { if (xtraPixW <= visits) { if (alternateW) { this.clip.right--; xtraPixW--; } alternateW = !alternateW; } else { this.clip.right--; xtraPixW--; } } this.clip.right -= incrementW; if (xtraPixH > 0) { if (xtraPixH <= visits) { if (alternateH) { this.clip.top++; xtraPixH--; } alternateH = !alternateH; } else { this.clip.top++; xtraPixH--; } } this.clip.top += incrementH; visits--; if (this.clip.height <= 0 && whichEl.clip.width <= 0) { clearInterval(transTimer); this.hideIt(); } } /* transition 18 - STRIPS LEFT UP: diagonal wipe not possible; box-in to top-left corner substituted; element transition from visible to hidden; */ function eighteen(){ if (xtraPixW > 0) { if (xtraPixW <= visits) { if (alternateW) { this.clip.right--; xtraPixW--; } alternateW = !alternateW; } else { this.clip.right--; xtraPixW--; } } this.clip.right -= incrementW; if (xtraPixH > 0) { if (xtraPixH <= visits) { if (alternateH) { this.clip.bottom--; xtraPixH--; } alternateH = !alternateH; } else { this.clip.bottom--; xtraPixH--; } } this.clip.bottom -= incrementH; visits--; if (this.clip.height <= 0 && whichEl.clip.width<=0) { clearInterval(transTimer); this.hideIt(); } } /* transition 19 - STRIPS RIGHT DOWN: diagonal wipe not possible; box-in to bottom-right corner substituted; element transition from visible to hidden; */ function nineteen(){ if (xtraPixW > 0) { if (xtraPixW <= visits) { if (alternateW) { this.clip.left++; xtraPixW--; } alternateW = !alternateW; } else { this.clip.left++; xtraPixW--; } } this.clip.left += incrementW; if (xtraPixH > 0) { if (xtraPixH <= visits) { if (alternateH) { this.clip.top++; xtraPixH--; } alternateH = !alternateH; } else { this.clip.top++; xtraPixH--; } } this.clip.top += incrementH; visits--; if (this.clip.height <= 0 && whichEl.clip.width<=0) { clearInterval(transTimer); this.hideIt(); } } /* transition 20 - STRIPS RIGHT UP: diagonal wipe not possible; box-in to top-right corner substituted; element transition from visible to hidden; */ function twenty(){ if (xtraPixW > 0) { if (xtraPixW <= visits) { if (alternateW) { this.clip.left++; xtraPixW--; } alternateW = !alternateW; } else { this.clip.left++; xtraPixW--; } } this.clip.left += incrementW; if (xtraPixH > 0) { if (xtraPixH <= visits) { if (alternateH) { this.clip.bottom--; xtraPixH--; } alternateH = !alternateH; } else { this.clip.bottom--; xtraPixH--; } } this.clip.bottom -= incrementH; visits--; if (this.clip.height <= 0 && whichEl.clip.width<=0) { clearInterval(transTimer); this.hideIt(); } } /* transition 23 - RANDOM: transitions 1, 3, 14 & 16 (hidden to visible) excluded from random selection element transition from visible to hidden only; */ function twentythree(dur){ newTrans = getRandNums(0,22); doTransNS(whichEl,newTrans,dur); } function getRandNums(from,to){ temp = parseInt((Math.random() * (to-from)) + (from)); while (isNaN(temp)) { temp = parseInt((Math.random() * (to - from)) + (from)); } for (i=0; i<arHidden.length; i++) { if (temp == arHidden[i]) { temp = parseInt((Math.random() * (to - from)) + (from)); while (isNaN(temp)) { temp = parseInt((Math.random() * (to - from)) + (from)); } i=0; } } return temp } function hideIt() { this.visibility = "hide"; this.clip.left = this.origLeft; this.clip.top = this.origTop; this.clip.right = this.origRight; this.clip.bottom = this.origBottom; }
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: Apr. 28, 1998
Revised: Apr. 28, 1998
URL: https://www.webreference.com/dhtml/column19/allCode2.html