New JavaScript Mouse Events: The Rollover/Click Effect
![]() ![]() ![]() ![]() ![]() |
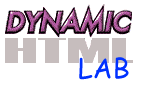
The Rollover-Click Effect:
using 4 event handlers with one button
A truly dynamic link button would not only have a click effect, but would show some sign of life when your mouse passed over it. That is, when it is active.
This is easily accomplished by appending the older onMouseOver and onMouseOut event handlers to our code.
Create New Images
We need two new images. One to represent the activated button (for onMouseOver) and one that will replace the old pressed button we had created. The pressed version should reflect the appearance of the activated button, not the regular, inactive button:
active button:
and more appropriate pressed button:
Combine all event handlers
We achieve our effect by appending these two code snippets to our <A> tag:onMouseDown = "document.images['imBut'].src='imButdown.gif'" onMouseUp = "document.images['imBut'].src='imButover.gif'"
Notice that onMouseUp inserts the active image. Our button is still active, even though we pressed it, because our mouse is still hovering over it.
Code
<A HREF="yourlinkgoeshere.html" onMouseOver = "document.images['imBut'].src='imButover.gif'" onMouseOut = "document.images['imBut'].src='imButup.gif'" onMouseDown = "document.images['imBut'].src='imButdown.gif'" onMouseUp = "document.images['imBut'].src='imButover.gif'"> <IMG NAME="imBut" SRC="imButup.gif" WIDTH=62 HEIGHT=28 BORDER=0></A>
Good Housekeeping: Using function calls
If we have more than one image on a page that warrants our effect, our event handlers should be made to call functions that perform the image swapping.
function rollOver(imName,over) { if (over) { document.images[imName].src = imName + "over.gif" } else { document.images[imName].src = imName + "up.gif" } } function rollPress(imName,down) { if (down) { document.images[imName].src = imName + "down.gif" } else { document.images[imName].src = imName + "over.gif" } }
Each of the functions is passed two arguments. The first, a string, is the name of the image that will have the effect applied to it. The second is a boolean to toggle which image src is inserted.
Now modify the <A> tag in the document to read:
<A HREF="yourlinkgoeshere.html" onMouseOver = "rollOver('imBut',true)" onMouseOut = "rollOver('imBut',false)" onMouseDown = "rollPress('imBut',true)" onMouseUp = "rollPress('imBut',false)"> <IMG NAME="imBut" SRC="imButup.gif" WIDTH=62 HEIGHT=28 BORDER=0></A>
onClick: The Fifth Wheel for Backward Compatibility
If you've been following along, using Navigator 3, and pressing buttons whenever you read "Click Me!", you may be mumbling: "Wait a minute! I'm not using Communicator and yet the buttons seem to press anyway."
Well, we've been faking it for compatibility. Read on.
We mentioned at the onset that onMouseDown and onMouseUp are just the two components of onClick. Recognized by all JS-enabled browsers, onClick can help us simulate a pressed button for those browsers that at least understand the Image object (NN3).
function rollClick(imName) { document.images[imName].src = imName + "down.gif"; dummy = setTimeout("document.images[imName].src = \"" + imName + "over.gif\"",100); }
In this script we replace our image with the down version when the link is clicked, then use the setTimeout method to bring back the over version 100 milliseconds later. Of course, the function is not called until the mouse button has returned to the up position, that is, clicked, but it still provides for a useful effect.
WARNING! Compatibility probs
We still have a problem, however. Remember:
Netscape Navigator 2.xx and Microsoft Internet Explorer 3.xx recognize only onMouseOver and do not have an Image object. (JavaScript 1.0)
Navigator 3.xx recognizes onMouseOver and onMouseOut and has an Image object. (JavaScript 1.1)
Navigator 4.xx and Explorer 4 recognize all four event handlers and have an Image object. (JavaScript 1.2)
Easy, you say, just use LANGUAGE="JavaScript1.x" in the <SCRIPT> tag and serve up the appropriate functions. This, although, a good solution, would work only for Netscape. The previous version of this column suggested exactly that. But, if we want to appease all versions of both browsers, we should discuss some compatibility issues.
Produced by Peter Belesis and
All Rights Reserved. Legal Notices.Created: 07/23/97
Revised: 09/28/97
URL: https://www.webreference.com/dhtml/column1/foursome.html