Getting Started with Silverlight | 2
[previous] [next]
Getting Started with Silverlight
Embedding the Silverlight Control Manually
Silverlight, just like Adobe Flash, is a web browser add-on. It's a pair of componentsÂone for Internet Explorer (an ActiveX control), and one for all other supported browsers (a Netscape plug-in)Âbut this is an invisible implementation detail to make things "just work" regardless of the host browser. The standard way for web pages to take advantage of an add-onÂwhether Silverlight, Flash, or anotherÂis with the OBJECT HTML element.
Listing 1.1 contains a simple web page for a fictional "Great Estates" housing development that embeds a Silverlight logo at the top using the OBJECT element.
LISTING 1.1 A Web Page with Embedded Silverlight Content
The id, width, and height attributes on the OBJECT element work the same way as on elements such as DIV, TABLE, and so on. For example, width and height can be specified in absolute pixel values or as a percentage. The type attribute refers to the MIME type of the add-on content. The Silverlight add-on is invoked by the host browser for any content of type application/x-silverlight.
The Silverlight add-on supports several custom parameters, covered later in the "Understanding Your Hosting Options" section. In this example, the background parameter is set to fill the 390x100 region with the color yellow, and the source parameter is pointing to a separate XAML file containing the content to be rendered on top of the yellow background. This XAML file, Chapter1.xaml, is shown in Listing 1.2.
LISTING 1.2 Chapter1.xamlÂA XAML File Containing a Logo
This XAML file defines a logo containing two lines of text, some vector artwork, and even a live video cropped by a circle! Don't worry about the syntax of the XAML file for now. The next chapter covers everything you need to know about XAML syntax, and the various Silverlight elements (Canvas, MediaElement, Ellipse, and so on) are covered throughout the remainder of the book.
Figure 1.1 displays the web page defined by Listings 1.1 and 1.2. Most web pages probably would make the Silverlight content blend in better by giving the OBJECT element a matching background, but for this example, the yellow background helps to highlight the area of the page rendered by Silverlight.
Of course, the Great Estates web page only resembles what's shown in Figure 1.1 if the viewer has the Silverlight add-on installed. Without the add-on, the page looks similar to Figure 1.2 (depending on which browser you use).
WARNING: HTML and CSS fonts, colors, and more are not inherited by Silverlight content! The fonts, colors, and other visual aspects of Silverlight content are completely indepen-dent from any other settings on the page. If you want to apply different themes to your Silverlight content, you'll need to employ a custom mechanism to make this happen.
Fortunately, there's a relatively easy solution for giving users who don't have the add-on a reasonable experience. If you place content directly inside the OBJECT element, browsers will render that content in the case of failure. Therefore, the OBJECT element in Listing 1.1 could be updated as follows to downgrade the logo to a simple image for viewers without Silverlight:
The logo in logo.png could look identical to the Silverlight logo shown in Figure 1.1, except that the live video would be a static image instead. If you don't want to create a downgraded version of your Silverlight content, you could always notify the user and help her install the Silverlight add-on:
Unfortunately, Apple's Safari web browser doesn't currently support the OBJECT element. Instead, you must use an element called EMBED, which also happens to work in Internet Explorer and Firefox. Listing 1.3 contains this update to Listing 1.1 in order to work on Safari as well.
LISTING 1.3 Embedding Silverlight Content Using EMBED Instead of OBJECT
Besides the different element name (EMBED versus OBJECT), the only other difference is that the custom parameters are specified as attributes of the EMBED element rather than as child elements. Alternative content (for when the embedding fails) can be specified with a separate NOEMBED element. The result from using EMBED looks the same as Figure 1.1 (at least the Silverlight content), as seen in Figure 1.3.
Using EMBED is the simplest way to get your content rendered in all supported browsers, despite the fact that OBJECT is preferred for Internet Explorer and Firefox.
Letting Silverlight.js Handle the Dirty Work
Embedding Silverlight content manually with an OBJECT or EMBED element has a number of issues. There's the concern about browser differences (although that can be avoided by always sticking to EMBED). Most importantly, it would be a fair amount of work to properly handle Silverlight detection. For example, although placing a download link as alternative content inside the OBJECT element (or using a NOEMBED element) seems simple enough, it doesn't behave appropriately if somebody has the wrong version of Silverlight installed. If a web page contains Silverlight content that uses future features unavailable in 1.0, viewers with 1.0 installed will not see the alternative content. Instead, the Silverlight 1.0 add-on will attempt to render the content and will fail.
Microsoft would be making a huge mistake if they asked everyone to do the appropriate version detection work on their own. The code involved is not straightforward, and version detection logicÂfor any softwareÂis notorious for being done incorrectly. (As silly as it sounds, someone might write logic that behaves properly for version numbers such as 1.0 and 1.1, but would fail years later when version 4.0 appears.) Sure enough, the Silverlight Software Development Kit (Silverlight SDK) provides a JavaScript file called Silverlight.js that defines a simple JavaScript function handling everything from injecting an appropriate OBJECT or EMBED element into an HTML document to checking if the right version of Silverlight is installed, and then directing the viewer to the appropriate place to install it if it isn't. You should always use the functionality in Silverlight.js (discussed in this section) rather than directly using OBJECT or EMBED unless your content must appear in an environment where JavaScript is not allowed.
Silverlight.createObject
The simple function exposed by Silverlight.js is Silverlight.createObject. Here is how createObject could be called in JavaScript to generate an OBJECT/EMBED element as shown in Listings 1.1 and 1.3:
The first parameter becomes the source value for the dynamically generated OBJECT or EMBED element, and the third parameter becomes its id. The second parameter can be an existing HTML element to contain the new OBJECT or EMBED element. In this example, the standard document.getElementById function is used to retrieve an element from the page via its HTML id (placeholder), but you could also pass document.body if you want to append the new element directly to the page's body.
The fourth and fifth parameters to createObject are associative arrays of properties and events, respectively, supported by the Silverlight add-on. The properties array is a mix of values that either alter the logic inside Silverlight.js (such as version), are applied directly to the OBJECT or EMBED element (such as width and height), or are applied as PARAM element children when the OBJECT element is used (such as background). The various properties (and events) are covered in the upcoming "Understanding Your Hosting Options" section. The only new property shown here is version, which should simply be set to the version of Silverlight you're targeting (1.0).
Tip: If you pass null for the parent HTML element, createObject returns a string containing the OBJECT or EMBED element that would have otherwise been added to the parent. This gives you some flexibility for morphing the element or otherwise customiz-ing how it is added to your page.
Tip: The createObject function has sixth and seventh (optional) parameters that can both be used to attach custom data to the Silverlight control. For example, if you set the sixth para-meter (initParams) to the string "custom", the dynamically generated OBJECT element would have the following additional child:
With this in place, you could write JavaScript that retrieves this value with standard DOM func-tions for traversing the tree of HTML elements or with a simple Silverlight-specific property called InitParams explained toward the end of this chapter. If you set the seventh parameter (context) to any object, that object will be passed as a parameter to the control's onLoad event handler (covered later in this chapter). This context functionality is specific to Silverlight.js and, unlike initParams, cannot be accomplished with a PARAM element in HTML. The capabilities provided by these two mechanisms are simply additional ways to communi-cate information between JavaScript files that might be developed as separate components.
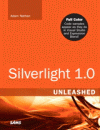
Printed with permission from Pearson Education from the book Silverlight 1.0 Unleashed written by Adam Nathan.
[previous] [next]
URL: