Working With Forms in PHP / Page 5
[previous]
Working With Forms (con't)
#31: Double-Checking a Credit Card's Expiration Date
When you accept a credit card, you'll need to know whether it has expired. In your HTML, it's best to create a drop-down menu that allows customers to choose their card's expiration date in order to avoid ambiguity in date formats:
Now that you have a form for entering an expiration date, you need to validate the data sent by it.
To check a credit card expiration date, all you have to do is make sure that the date falls between the current date and some date in the future (this function uses 10 years). The best tools for that task are described in Chapter 6, so consider this a sneak preview.
The only trick here is that a credit card becomes invalid after the last day of the month in its expiration date. That is, if a card's expiration date was 06/2005, it actually stopped working on July 1, 2005. Thus, we have to add a month to the given date. This can be a pain because it can also set the actual target date ahead a year, but as you will learn in Chapter 6, the mktime()
function that we're using here to compute the expiration timestamp automatically compensates for month numbers that are out of range. After computing the expiration timestamp, all you need are the current and maximum timestamps, and validating the expiration time boils down to a pair of simple comparisons.
Using the Script
#32: Checking Valid Email Addresses
Customers enter all sorts of weird data into email form fields. The script in this section verifies that an email address mostly follows the rules outlined in RFC 2822. This won't prevent someone from entering a false (but RFC-compliant) email address such as [email protected], but it will catch some typos.
NOTE: If having a valid email address is critical, you need to require user accounts that are activated by links sent only via email. You'll see how to do this in "#65: Using Email to Verify User Accounts" on page 124. This is a fairly extreme measure; if you want more people to share their addresses with you, simply tell users that you won't spam them (and make good on that promise).
This script utilizes a regular expression to check whether the given email uses proper email characters (alphabetical, dots, dashes, slashes, and so on), an @ sign in the middle, and at least one dot-something on the end. You can read more on regular expressions in "#39: Regular Expressions" on page 69.
#33: Checking American Phone Numbers
As with email addresses, there's no way to make sure a telephone number is valid outside of making a real telephone call. However, you can validate the number of digits and put it into standard format. The following function returns a pure 10-digit phone number if the number given is 10 digits or 11 digits starting with 1. If the number does not conform, the return value is false.
This script shows the power of regular expressions combined with standard string functions. The key is to first throw out any character that's not a digit—a perfect task for the preg_replace()
function. Once you know that you have nothing but digits in a string, you can simply examine the string length to determine the number of digits, and the rest practically writes itself.
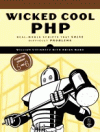
Excerpted from Wicked Cool PHP, by William Steinmetz with Brian Ward. February 2008, ISBN 1593271735. Published by No Starch Press
[previous]
URL: