Working With Forms in PHP / Page 2
[previous] [next]
Working With Forms (con't)
#25: Fetching Form Variables Consistently and Safely
You should pull form data from predefined server variables. All data passed on to your web page via a posted form is automatically stored in a large array called $_POST
, and all GET data is stored in a large array called $_GET
. File upload information is stored in a special array called $_FILES
(see "#54: Uploading Images to a Directory" on page 97 for more information on files). In addition, there is a combined variable called $_REQUEST
.
To access the username field from a POST method form, use $_POST['username']
. Use $_GET['username']
if the username is in the URL. If you don't care where the value came from, use $_REQUEST['username']
.
$_REQUEST
is a union of the $_GET
, $_POST
, and $_COOKIE
arrays. If you have two or more values of the same parameter name, be careful of which one PHP uses. The default order is cookie, POST, then GET.
There has been some debate on how safe $_REQUEST
is, but there shouldn't be. Because all of its sources come from the outside world (the user's browser), you need to verify everything in this array that you plan to use, just as you would with the other predefined arrays. The only problems you might have are confusing bugs that might pop up as a result of cookies being included.
#26: Trimming Excess Whitespace
Excess whitespace is a constant problem when working with form data. The trim() function is usually the first tool a programmer turns to, because it removes any excess spaces from the beginning or end of a string. For example, " Wicked Cool PHP " becomes "Wicked Cool PHP." In fact, it's so handy that you may find yourself using it on almost every available piece of user-inputted, non-array data:
But sometimes you have excessive whitespace inside a string—when someone may be cutting and copying information from an email, for instance. In that case, you can replace multiple spaces and other whitespace with a single space by using the preg_replace()
function. The reg stands for regular expression, a powerful form of pattern matching that you will see several times in this chapter.
You'll find many uses for this script outside of form verification. It's great for cleaning up data that comes from other external sources.
#27: Importing Form Variables into an Array
One of the handiest tricks you can use in PHP is not actually a PHP trick but an HTML trick. When a user fills out a form, you'll frequently check the values of several checkboxes. For example, let's say you're taking a survey to see what sorts of movies your site's visitors like, and you'd like to automatically insert those values into a database called customer_preferences
. The hard way to do that is to give each checkbox a separate name on the HTML form, as shown here:
Unfortunately, when you process the form on the next page, you'll need a series of if/then
loops to check the data—one loop to check the value of $action
, one to check the value of $drama
, and so forth. Adding a new checkbox to the HTML form results in yet another if/then
loop to the processing page.
A great way to simplify this procedure is to store all of the checkbox values in a single array by adding []
after the name, like this:
When PHP gets the data from a form like this, it stores the checked values in a single array. You can loop through the array this way:
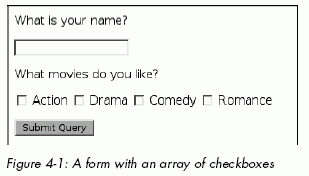
—
Not only does this technique work for checkboxes, but it's extremely handy for processing arbitrary numbers of rows. For example, let's say we have a shopping menu where we want to show all the items in a given category. Although we may not know how many items will be in a category, the customer should be able to enter a quantity into a text box for all items he wants to buy and add all of the items with a single click. The menu would look like Figure 4-1.
Let's access product name and ID data in the product_info MySQL table described in the appendix to build the form as follows:
To process the form, you need to examine the two arrays passed to the processing script—one array containing all of the product IDs ($product_id
) and another containing the corresponding values from the quantity text boxes ($quantity
). It doesn't matter how many items are displayed on the page; $product_id[123]
contains the product ID for the 123rd item displayed and $quantity[123]
holds the number the customer entered into the corresponding text box.
The processing script addtocart.php
is as follows:
As you can see, this script depends wholly on using the index from the $quantity
array ($key
) for the $product_id
array.
[previous] [next]
URL: