Object-Oriented JavaScript | Page 3
[next] [next]
Object-Oriented JavaScript
JavaScript Functions
A simple fact that was highlighted in the previous chapter, but that is often overlooked, is key to understanding how objects in JavaScript work: code that doesn't belong to a function is executed when it's read by the JavaScript interpreter, while code that belongs to a function is only executed when that function is called.
Take the following JavaScript code that you created in the first exercise of Chapter 2:
|
This code resides in a file named JavaScriptDom.js
, which is referenced from an HTML file (JavaScriptDom.html
in the exercise), but it could have been included directly in a <script>
tag of the HTML file. How it's stored is irrelevant; what does matter is that all that code is executed when it's read by the interpreter. If it was included in a function it would only execute if the function is called explicitly, as is this example:
This code has the same output as the previous version of the code, but it is only because the ShowHelloWorld()
function is called that will display "Goodnight, world!" or "Hello, world!" depending on the hour of the day. Without that function call, the JavaScript interpreter would take note of the existence of a function named ShowHelloWorld()
, but would not execute it.
Functions as Variables
In JavaScript, functions are first-class objects. This means that a function is regarded as a data type whose values can be saved in local variables, passed as parameters, and so on. For example, when defining a function, you can assign it to a variable, and then call the function through this variable. Take this example:
When storing a piece of code as a variable, as in this example, it can make sense to create it as an anonymous function—which is, a function without a name. You do this by simply omitting to specify a function name when creating it:
Anonymous functions will come in handy in many circumstances when you need to pass an executable piece of code that you don't intend to reuse anywhere else, as parameter to a function.
Let's see how we can send functions as parameters. Instead of sending a numeric hour to DisplayGreeting()
, we can send a function that in turn returns the current hour. To demonstrate this, we create a function named GetCurrentHour()
, and send it as parameter to DisplayGreeting()
. DisplayGreeting()
needs to be modified to reflect that its new parameter is a function—it should be referenced by appending parentheses to its name. Here's how:
This code can be tested online. The output should resemble Figure 3-1.
[ .NET languages such as C# and VB.NET support similar functionality through the concept of delegates. A delegate is a data type that represents a reference to a function. An instance of a delegate represents a function instance, and it can be passed as a parameter to methods that need to execute that function. Delegates are the technical means used by .NET to implement event-handling. C# 2.0 added support for anonymous methods, which behave similarly to JavaScript anonymous functions. ]
Anonymous Functions
A nonymous functions can be created adhoc and used instead of a named function. Although this can hinder readability when the function is more complex, you can do this if you don't intend to reuse a function's code. In the following example we pass such an anonymous function to DisplayGreeting()
, instead of passing GetCurrentHour()
:
This syntax is sure to look strange if this is the first time you have worked with anonymous functions. You can compact it on a single line if it helps understanding it better:
This code can be tested online.
Inner Functions and JavaScript Closures
JavaScript functions implement the concept of closures, which are functions that are defined inside other functions, and use contextual data from the parent functions to execute. You can find a complete and technically accurate definition of closures at https://en.wikipedia.org/wiki/Closure_(computer_science).
In JavaScript a function can be regarded as a named block of code that you can execute, but it can also be used as a data member inside another function, in which case it is referred to as an inner functions. In other words, a JavaScript function can contain other functions.
Say that we want to upgrade the initial ShowHelloWorld()
function by separating the code that displays the greeting message into a separate function inside ShowHelloWorld()
. This is a possible implementation, and the output continues to be the same as before:
Here, we created a function named DisplayGreeting()
inside ShowHelloWorld()
, which displays a greeting message depending on the hour parameter it receives. The execution rules apply here as well. This new function needs to be called explicitly from its parent function in order to execute.
This code can be tested online.
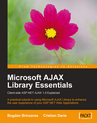
[This is an escerpt from the book, Microsoft AJAX Library Essentials: Client-side ASP.NET AJAX 1.0 Explained, by Cristian Darie, Bogdan Brinzarea . Published by Packt Publishing Ltd., 2007
[next] [next]
URL: