The JavaScript Diaries: Part 5 - Page 8
[previous]
The JavaScript Diaries: Part 5
The while
statement
A while
statement is good for short comparisons. It will continue to repeat until the comparison is false. Unlike the for
statement, it does not create its own variable. It must use an existing one from the main script. Its format is:
while (condition) { statements }
With this type of loop it's important to remember that the initial value of the variable must be declared before the loop and the value must be adjusted within the loop, otherwise it will repeat indefinitely. We can use the while
statement to do what we did with the for
statement previously:
var jsNot=1; while (jsNot<11) { document.write(jsNot + ".JavaScript is not Java!<br>"); jsNot+=1; }
Notice that the variable is declared for the loop prior to its declaration;
the comparison statement is located after the while
declaration;
and the incremental statement is after the performed action. Without the incremental
statement, the script won't stop, it will go on indefinitely. (I know, I tried
it. Thanks to Firefox, it detected it and asked me if I wanted to stop it. Nice
feature!)
The diagram below may be helpful.
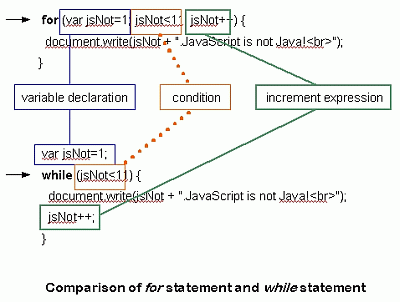
The do while
statement
This is different from the while
statement in that it will always execute the loop at least once, even if it's false. The comparison is made at the end of the statement. The format is:
do { statement } while (condition);
An example would be:
var i=1 do { document.write(i + "<br>") i++ } while (i <= 6);
In this statement, we first declared a variable named "i
" and initialized it with a value of 1. (The variable "i
" is generally used as a variable name for this kind of a counter.) Next, the statement will print out the number 1 to the Web page using the document.write
statement. Then the value of "i
" will be increased by 1 using the ++
increment operator. The last line, the while
portion of the statement, checks to see if the variable "i
" is less than or equal to (<=
) the number 6. If the statement is true (the variable "i
" is less than or equal to (<=
) the number 6), then the loop will start over again. If the statement is false, the loop will end.
Wrap-Up
That covers our study of conditional statements and loops. Hopefully you have gained enough insight to start creating some of your own scripts, albeit very simple ones. Perhaps you can even modify some existing ones to better suit your needs. Whatever the case, keep on scripting! See you next time.
Continue on to "The JavaScript Diaries: Part 6"
[previous]
Created: June 10, 2005
URL: