Google Web Toolkit Solutions: Cool & Useful Stuff
Google Web Toolkit Solutions: Cool & Useful Stuff
What This Short Cut Covers
The Google Web Toolkit (GWT) is a cutting-edge UI framework for Java developers, which lets you create rich, interactive user interfaces using familiar idioms from Java's Abstract Window Toolkit (AWT), Swing, and the Eclipse Foundation's SWT. If you've used any of those frameworks in the past, you're already halfway up the GWT learning curve.
This short cut assumes that you have already installed GWT and have experimented with its basic features. It also assumes that you're comfortable with techniques like implementing event listeners as anonymous inner classes and know how to construct applications using panels and widgets. Some of the more advanced aspects of the GWT are explored in this short cut using two applications: an address book and a Yahoo! trip viewer.
Both applications use remote procedure calls to access information on the server or an online web service. The Yahoo! Trips application also shows how you can incorporate Scriptaculous, a powerful JavaScript toolkit, to apply a useful effect for displaying results. Other cool and useful techniques, including how to implement drag and drop and how to integrate with a database using Hibernate are demonstrated. Since you'll eventually want to move your GWT application to a servlet container such as Tomcat or Resin, the process of deploying a GWT application to Tomcat with Ant is also covered. Lastly, this short cut shows how to use popup panels and deferred commands to provide a much more interactive user interface.
TIP: Render unto client ...
The GWT is sometimes criticized for not fully supporting Java on the client. Remember two things, however: first, some Java classes don't make sense in a browser, for example, sockets or files; second, if you do need the full power of Java, you have RPCs to do your heavy lifting.
Incorporate JavaScript and JavaScript Toolkits
The GWT exists so that Java developers can easily create Ajax-enabled applications without resorting to JavaScript, and for the most part, you can get by with very little knowledge of JavaScript when you use the GWT to implement your web applications.
On the other hand, however, there are a number of useful JavaScript toolkitsÂsuch as Prototype, Dojo, Scriptaculous, Rico, and so forthÂthat can greatly increase your productivity and make your applications much more usable. You may also, from time to time, have the urge to dip into some JavaScript yourself.
In the Yahoo! Trips application, we use Scriptaculous to apply a grow effect to a panel that contains the results of our search. The effect is shown in Figure 4.
The GWT makes ingenious use of Java's native methods with something called the JavaScript Native Interface. You declare native methods with commented-out bodies that contain JavaScript. When those native methods are compiled, the GWT incorporates the commented JavaScript. Here's an example of such a native method:
Figure 4: Using the Scriptaculous grow effect
It's vital that you implement JSNI methods exactly as outlined above. The JavaScript can be any valid JavaScript code, but the surrounding native method declaration must follow the preceding syntax. For example, if you change the preceding JSNI method to this (I left off the dashes before and after the braces) ...
... the GWT will not load your application. Instead, when you run the application in hosted mode, the GWT will popup a dialog that says "Failed to load module . . . ." Also, in the Development Shell window you, will be presented with the following ugliness:
Figure 5: The GWT may not load your application if you have a JSNI syntax error
JSNI methods have another special requirement: you must access the window and document JavaScript elements through objects named $wnd
and $doc
, respectively.
Incorporate JavaScript Toolkits
JavaScript frameworks such as Prototype and Scriptaculous offer a great deal of functionality that you might like to use in your GWT applications. For example, we could write a JSNI method to perform a Scriptaculous effect:
Scriptaculous creates an object named Effect
and adds it to the window object. The preceding code accesses that object to perform an effect.
There are two ways to include JavaScript code in a GWT application. The simplest is to include script elements in your application's HTML file, like this:
The preceding script elements include Prototype and the Effects library from Scriptaculous.
PITFALL: Give <script>
elements an empty body
If you use a <script>
element to include your JavaScript in your application's HTML file, don't do this:
Instead, do this:
<script>
element, or your JavaScript will not be processed. Worse yet, if you don't provide an empty body in the Yahoo! Trips application, the GWT will not call your application's onModuleLoad
method, meaning the application won't work at all. That can lead to a lengthy and difficult debugging session.
PITFALL: Integrating Scriptaculous on the Mac
In the preceding code fragment, we did this:
That will work fine on the Windows distribution of the GWT, but on the Mac, the same code will result in a nasty exception. The workaround is to do this instead:
The preceding workaround on the Mac is necessary due to an issue with Safari and iframes. In effect, the preceding code forces Prototype to hand-copy the appropriate functions directly to the DOM element the function is passed.
Script Injection for Importing JavaScript
Adding script elements to your application's HTML file, as outlined in the preceding section, is one way to include JavaScript code in your application. The GWT provides an alternative mechanism for loading JavaScript files: You specify the JavaScript files that you want to load in your GWT configuration file. For example, Listing 1 shows the Yahoo! Trips application configuration file.
LISTING 1 com.acme.YahooTripsApplication.gwt.xml
The preceding JavaScript checks for the existence of certain JavaScript objectsÂPrototype's $ function and Scriptaculous's Effect objectÂas evidence that those libraries have loaded. When those objects are detected, the functions return true to indicate to the GWT that the libraries have been loaded.
PITFALL: Read this if you can't inject Scriptaculous
This won't work:
But this will:
You must load Scriptaculous files directly, as illustrated by the second code fragment above. Loading scriptaculous.js
with a request parameter to denote the library that you want to load—which is typically how Scriptaculous libraries are loaded—will not work with the GWT. The technical reasons have to do with a mismatch between the way GWT and Scriptaculous process script elements. But regardless of that mismatch, if you always include your Scriptaculous JavaScript files directly, you won't have a problem.
TIP: Import JavaScript in your module's configuration file
Notice that you have a choice of how you import JavaScript. You can either include it with a script element in your HTML page, or you can include it in your GWT configuration file. The former is more familiar, but the latter lets you develop modules that others can use without having to manually include the JavaScript that the module requires.
PITFALL: Go through these JavaScript objects
Don't forget the $wnd
and $doc
objects in your JavaScript! For JavaScript that you implement in native methods, you must access the JavaScript window object through $wnd
and the document object through $doc
. If you fail to do so for the applyEffect()
method from the preceding example, you will get the following exception (look in Development Shell window for that exception):
(The exception stacktrace is truncated here for brevity)
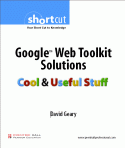
This excerpt is taken from Chapter 2 of the Digital Short Cut titled, Google Web Toolkit Solutions: Cool & Useful Stuff (not yet published), authored by David Geary, published by Prentice Hall Professional in January, 2007, ISBN 0131584650, Copyright 2007 Pearson Education, All rights reserved. For more information on Digital Short Cuts, please visit: https://www.informit.com/shortcuts or https://safari.informit.com/
URL: