Creating Responsive GUIs with Real-Time Validation | Page 5
[previous]
Creating Responsive GUIs with Real-Time Validation
Giving Validator an Extreme Ajax Makeover
The Validator application is one of the more immediately applicable examples in the book because intelligent data entry is a common need. The validatekit.js
file offers functions for common data types that are often used in data entry forms. The first way to expand upon the Validator example is to apply the validation functions to your own user-input forms. This is as easy as importing the validatekit.js
file into your pages via the following <script>
tag:
Then code the HTML elements for validation by calling the appropriate validation function and passing along the IDs of the HTML elements. As an example, if you wanted to validate the age of a person to make sure it is an integer number, you might create the following text box and help field:
You don't have to associate a style class with the help text, but it does make the user interface look a little better. Here's an example of a help style that you can use to dress up the help fields:
Keep in mind that this style must be placed in the head of the Web page, which is also where the <script>
tag you saw a moment ago must be placed. This style just makes the help text italicized and red. You can easily add on more styles or tweak these to get a different look.
The one problem with this age validator is that it doesn't account for the user entering an age that doesn't make sense. For example, because the validation only checks to see if the input is an integer, no help text is displayed if the user enters an age of -5 or 212. And until someone uncovers the fountain of youth, an age much over 100 can probably be considered invalid. You could account for negative ages by possibly creating a whole new validation function that only allows positive integers, but in this particular case a range validation makes more sense.
The age range validation is responsible for first checking that an input value is an integer, and then further checking to see if the integer is within an acceptable range, such as 1 to 120. Following is how you might put together a validation function to carry out this task:
Notice that this function first calls the validateInteger()
function to check and make sure the input is indeed a valid integer. There's no sense in moving on to the range check if the input isn't an integer. If the input is an integer, a range check is performed. The result of the range check is to simply set or clear text in the help control. The help control is checked for a null
value in the event that you forget or decide not to include a help control, in which case you don't want an error to occur.
Even if your JavaScript experience is shaky or nonexistent, you should be able to isolate the key part of the validateAge()
function and modify it to fit your needs. For example, you can change the range of the validation check by simply changing this line of code:
Let's say you want to validate an NFL (National Football League) quarterback rating, which must be in the range 0 to 158. Here's how you would change the range-testing line of code:
Note: Please don't ask why an NFL quarterback rating is in the range 0 to 158 (actually 158.33), as opposed to something more logical such as 0 to 10. It's a tricky calculation that factors in variables such as pass completion percentage, yards per attempt, touchdowns per attempt, etc. Unless you're a total (American) football nerd, just accept the range and move on. Otherwise, feel free to learn more at https://en.wikipedia.org/wiki/Passer_rating.
Hopefully you can see that crafting your own validation functions out of the base set I've provided isn't terribly difficult.
It's also possible to extend the Ajax-style validation employed by the Validator application in looking up the city and state of a ZIP code. For example, a popular Ajax validation is to check a database of user names to ensure uniqueness when registering a new user. In this case, you would issue an Ajax request to your server script that passes along the user name entered, and the server script then checks the name against a database of existing user names to see if it is unique. The response could be as simple as "yes" or "no" for the uniqueness validation. So the client just needs to look at this result and display a help message accordingly.
The point to this makeover discussion is that I've really only exposed the tip of the iceberg in terms of data validation and Ajax. It's up to you to take what you've learned and the code I've provided to further expand on the validation techniques as needed.
Summary
Although this book presents lots of interesting Ajax applications, some with impressive visuals and live data feeds, from a purely practical perspective few of them are as immediately handy as the Validator application. The application has a very basic user interface, but it's the usability that shines here. The help text that appears when invalid data is entered is subtle but compelling. And consider that this help information appears immediately without you having to submit any data— this is the "Ajax" way of doing things, even when an Ajax server request isn't explicitly being made. Of course, the Validator application does involve an Ajax server request for obtaining the city and state for a ZIP code, but the fact remains that the application promotes an "Ajax" feel throughout, even when the validation is taking place solely on the client.
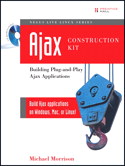
This chapter is excerpted from the book titled, Ajax Construction Kit: Building Plug-and-Play Ajax Applications, authored by Michael Morrison, published by < http:="">Prentice Hall, © 2007 Pearson Education, Prentice Hall PTR. All rights reserved.
[previous]
URL: