Web Services, Part V: XML and XSLT Programming: Defining and Reusing Variables - Doc JavaScript
![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() ![]() |
Web Services, Part V: XML and XSLT Programming
Defining and Reusing Variables
XSLT supports variables. You can set a variable in one place and reuse it somewhere else. Use variables to make your code more flexible and well-organized. You define a variable with the xsl:param
tag. You position this tag in the front section of your script, before the HEAD
statament. Here is an example:
<xsl:param name="forecast" select="50000"/>
And here is an example for the whole front section:
<?xml version="1.0"?> <xsl:stylesheet xmlns:xsl=" https://www.w3.org/1999/XSL/Transform" version="1.0"> <xsl:output method="html"/> <xsl:param name="forecast" select="50000"/> <xsl:template match="/"> <HTML> ... ... ...
You use a variable by prefixing its name with a $
sign. For example:
$forecast
Let's add a new column to our MyDVD Rental Store
's report. This column will show the sales forecast for each month. Let's also fix the forecast at 50,000 per month, for all months. It makes sense to define a variable called forecast
and set it to 50,000
. During printing, we don't have to repeat the number three times -- we use the variable forecast
.
To add the column header, Forecast, is straightforward:
<TH>Forecast</TH>
To add the forecast number for a single month can be done like this:
<td style="text-align:right;font-weight:bold"> <xsl:value-of select="format-number($forecast, '###,###')"/> </td>
Here is the XSLT file that converts the MyDVD XML file (try it)(view it):
<?xml version="1.0"?> <xsl:stylesheet xmlns:xsl=" https://www.w3.org/1999/XSL/Transform" version="1.0"> <xsl:output method="html"/> <xsl:param name="forecast" select="50000"/> <xsl:template match="/"> <HTML> <HEAD> <TITLE><xsl:value-of select="//summary/heading"/></TITLE> </HEAD> <BODY> <H1><xsl:value-of select="//summary/heading"/></H1> <H2><xsl:value-of select="//summary/subhead"/></H2> <P><xsl:value-of select="//summary/description"/></P> <TABLE> <TR> <TH>Month\Week</TH> <xsl:for-each select="//data/month[1]/week"> <TH>W<xsl:value-of select="@number"/></TH> </xsl:for-each> <TH>Total</TH> <TH>Forecast</TH> </TR> <xsl:for-each select="//data/month"> <tr> <th style="text-align:left"><xsl:value-of select="name"/></th> <xsl:for-each select="week"> <td style="text-align:right"> <xsl:value-of select="format-number(@dvds_rented, '###,###')"/> </td> </xsl:for-each> <td style="text-align:right;font-weight:bold"> <xsl:value-of select="format-number(sum(week/@dvds_rented), '###,###')"/> </td> <td style="text-align:right;font-weight:bold"> <xsl:value-of select="format-number($forecast, '###,###')"/> </td> </tr> </xsl:for-each> </TABLE> </BODY> </HTML> </xsl:template> </xsl:stylesheet>
Here is the output:
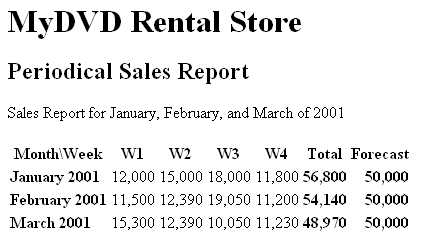
Next: How to implement conditional statements in XSLT
Produced by Yehuda Shiran and Tomer Shiran
All Rights Reserved. Legal Notices.
Created: December 31, 2001
Revised: December 31, 2001
URL: https://www.webreference.com/js/column100/8.html